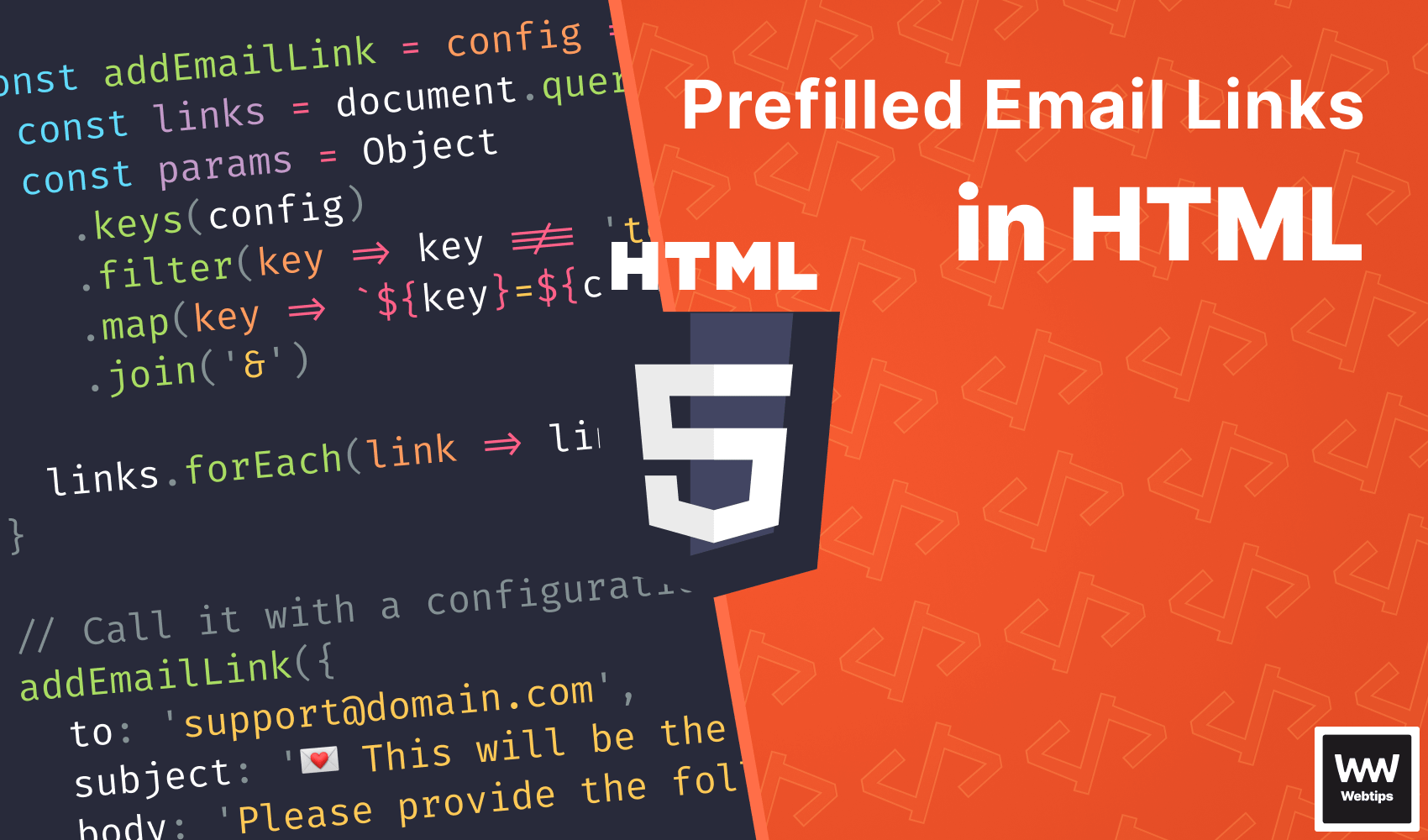
How to Create Prefilled Email Links in HTML
When it comes to sending emails in web applications, a common approach is to build a custom contact form that handles user input, validation, and the sending of messages. However, for simple use cases, there are easier solutions.
In this tutorial, we'll take a quick look at how you can use HTML anchors to trigger default email clients with prefilled inputs.
How to Create Email Links
To create email links in HTML, we need to use the <a>
tag with the href
attribute starting with the special mailto:
syntax pointing to the recipient:
<a href="mailto:support@domain.com">Get in touch</a>
Clicking on the above link will open the default email client with the recipient being set to support@domain.com
. You can give it a try using the following link: link with mailto.
How to Add Subjects
The mailto
syntax can also be used to prefill the subject line using the subject parameter attached after the email address:
<a href="mailto:support@domain.com?subject=π This will be the subject">Get in touch</a>
The structure follows the conventional syntax of query parameters. You'll notice that we have spaces in the subject line. This will work fine for most modern email clients.
However, if you need to support old and outdated clients, you can replace spaces with %20
to ensure they're parsed correctly. To see how the above functionality will behave, you can click on this link.

How to Add Body
In case you want senders to follow a basic structure when sending emails to you, you can also specify the body using the body parameter in the URL:
<a href="mailto:support@domain.com
?subject=π This will be the subject
&body=Please provide the following details..."
>
Get in touch
</a>
Note that the mailto functionality has a limit of around 2000 characters, so keep the body as short as possible. Click on this link if you would like to test the above code.
In case you want to insert new lines, you can use %0D%0A
, which is the URL-encoded version of a new line character.
How to Add CC and BCC
Last but not least, the mailto
syntax can also be used to add CC and BCC. Just like with the previous two attributes, this can be chained at the end of the URL using the cc
and bcc
parameters:
<a href="mailto:support@domain.com&cc=info@domain.com">Get in touch</a>
In case you need to provide multiple recipients, you can provide a comma-separated list for both parameters. For example, cc=support@mail.com, info@mail.com
. To test the cc
and bcc
parameters, you can use this link.
Create Email Links With JavaScript
When setting multiple parameters, it can become difficult to read and maintain an email link. We can make it more configurable with the help of JavaScript. The following function can help you dynamically create email links without the use of any libraries:
const addEmailLink = config => {
const links = document.querySelectorAll('[data-email-link]')
const params = Object
.keys(config)
.filter(key => key !== 'to')
.map(key => `${key}=${config[key]}`)
.join('&')
links.forEach(link => link.href = `mailto:${config.to}?${params}`)
}
// Call it with a configuration object in your application
addEmailLink({
to: 'support@domain.com',
subject: 'π This will be the subject',
body: 'Please provide the following details...'
})
To use the above code, call it with the configuration object. The to
parameter is required, and the rest are optional. To define an <a>
tag as an email link, you can attach a data-email-link
attribute to the element:
<a data-email-link>Get in touch</a>
The function will traverse through all elements flagged with the data-email-link
attribute and attach the proper href
attribute to the DOM elements using the configuration. The parameters are converted into a URL using the following process:
// #1: return the keys of the object as an array
// -> ['to', 'cc', 'bcc', ...]
Object.keys(config)
// #2: filter out the `to` property from the array
// -> ['cc', 'bcc', ...]
Object.keys(config).filter(key => key !== 'to')
// #3: map through the array and connect each key with its value
// -> ['cc=support@mail.com', 'bcc=info@mail.com']
Object.keys(config)
.filter(key => key !== 'to')
.map(key => `${key}=${config[key]}`)
// #4: Join the array elemnts into a string using `&`
// -> "cc=support@mail.com&bcc=info@mail.com"
Object.keys(config)
.filter(key => key !== 'to')
.map(key => `${key}=${config[key]}`)
.join('&')
Conclusion
In conclusion, the mailto
syntax with anchors is a versatile way to create email links without implementing a custom contact form. However, there are some considerations you need to keep in mind when using the mailto
attribute.
This will expose your email address and can potentially be picked up by spammers. If you need to keep the email address private, make sure you don't use the mailto
attribute. Nonetheless, mailto
provides a quick and easy way to accept emails through your website.
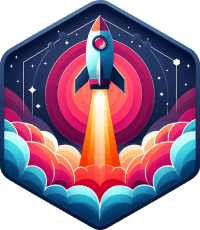
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: