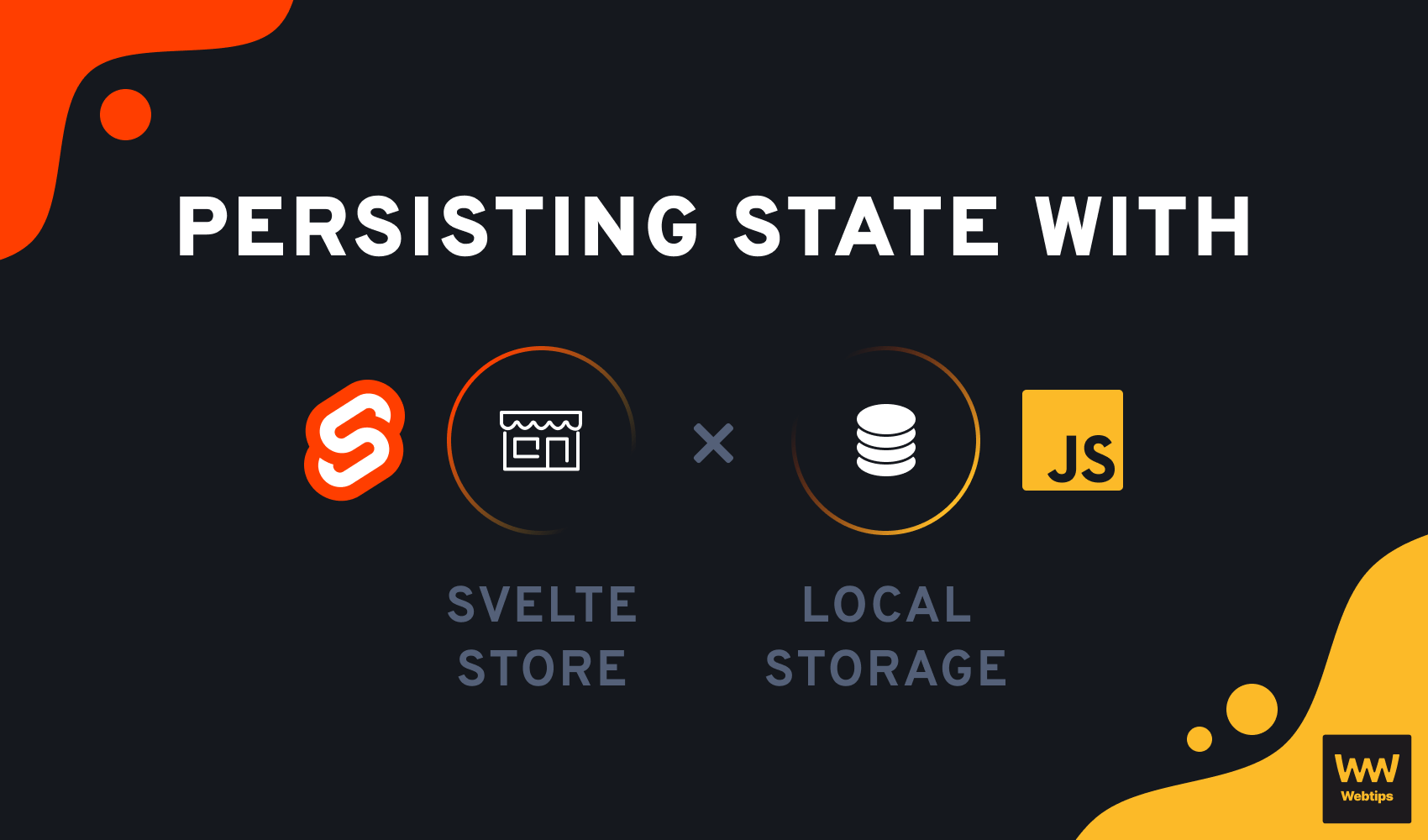
How to Save Writable Store in Svelte to Local Storage
Stores in Svelte are special objects that have a subscribe
method that allows interested parties to be notified whenever the store's value changes. A writeable
store also has an update
, and set
methods so that it can be modified.
The reason stores are a perfect fit for persisting state, is that they can be accessed anywhere, globally, from multiple unrelated components. This way, we are going to have a centralized place where we can store our application's state.
Connect the Writable Store To Local Storage
To connect a writable store in Svelte to local storage, we need two things; a file, let's call it state.js
and writable
imported from svelte/store
, as well as a reference to a local storage item, in this case, called "state":
import { writable, get } from 'svelte/store'
const localState = localStorage.getItem('state')
const initialState = {
users: []
}
if (!localState) {
// Set localStorage "state" to the "initialState"
}
const appState = localState ? JSON.parse(localState) : initialState
export const state = writable(appState)
export const update = callback => {
const updatedState = callback(get(state))
state.update(() => updatedState)
localStorage.setItem('state', JSON.stringify(updatedState))
}
Don't forget to use JSON.parse
and JSON.stringify
to correctly write and read local storage.
Letβs briefly discuss what this code actually does:
- First, we import the built-in
writable
store fromsvelte/store
. - Starting from line:4, our initial state will hold an empty
users
array. This is where you can set up all initial fields, such as auser
orsettings
object. - On line:12, we can use this initial state to set our
localState
in case we don't have any data yet. This will only be true for the very first use. - We export the state that we will access from other components (line:14).
- Below it, we also export an
update
function that we will use to update the state.
We are also missing a line between the if statement. Try to guess how to set the state
inside localStorage
to the initialState
variable.
For passing the correct state to the writable store (called appState
), we want two things to happen, based on whether we already have information in local storage or not:
- Use
localState
when it's available, meaning we already have data saved in local storage. - Use the
initialState
when no data is available inside local storage yet.
The update
function that we exported will update both the internal state of the application, as well as the data stored inside local storage. It will use a callback function and the built-in get
method of Svelte.
How to Read and Update the State
Now let's see how we can actually read and update our state. Since our state will reference a writable store, in order to get its value, we need to use the built-in get
function. Take a look at the following example of what we will get if we try to log the state with and without using the get
function:
<script>
import { get } from 'svelte/store'
import { state } from './state'
</script>
<!-- β This will output: {} -->
<pre>State without get: {JSON.stringify(state)}</pre>
<!-- βοΈ This will output: { users: [] } -->
<pre>State with get: {JSON.stringify(get(state))}</pre>
As we can see, without using get
, we get an empty object back, and not our actual state. This is why we need to use the built-in get
function. There is also another alternative, simpler way to get the value of a store in Svelte.
The $ symbol
<ul>
{#each $state.users as user}
<li>{user.id}: {user.name}</li>
{/each}
</ul>
Notice the dollar sign in front of the state. This is an alternative, simpler approach that we can use in place of the get
method to read values from our store. Now let's take a look at how to update our persistent state.
Updating the state
For updating the state, we need to import our update
method and pass a callback function to set the state. Take a look at the following example:
<script>
import { update } from './state'
const addUser = () => {
update(state => {
state.users.unshift({
id: state.users.length + 1,
name: 'John'
})
return state
})
}
</script>
Notice that at the end of our update
function, we need to return the state, as we expect it in our state.js
file. Anything we return from our update
function will be applied to our state and persisted through page reloads. As a reminder, this is how state.js
executes the callback function:
// The `updatedState` is then passed over to both the store and local storage
const updatedState = callback(get(state))

Conclusion
In conclusion, in order to persist state with Svelte stores using local storage, we need to read local storage and pass its value to a writable
store. To also update the store, we can use the update
method on the writeable store with a custom update
function that keeps track of both the writable store, as well as local storage to persist the state of our application.
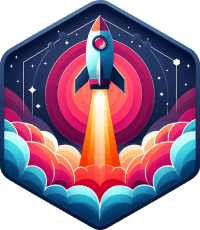
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: