How to Check Variable Types in TypeScript
To check variable types in TypeScript, use the typeof
operator which returns the type of a variable as a string. Take the following code as an example:
const variable1: string | number = '42'
const variable2: string | number = 42
// This will return "string"
console.log(typeof variable1)
// This will return "number"
console.log(typeof variable2)
// This is called typeguard
if (typeof variable1 === 'string') {
variable1.toLowerCase()
}
Using a type check to execute code is called a typeguard, and can ensure that you work with the correct type. For example, we don't have a toLowerCase
method on numbers, but we have it for strings. The typeof
operator can have the following return values:
typeof '1' // Returns "string"
typeof 1 // Returns "number"
typeof NaN // Returns "number"
typeof true // Returns "boolean"
typeof undefined // Returns "undefined"
typeof Symbol() // Returns "symbol"
typeof [] // Returns "object"
typeof null // Returns "object"
If you are interested in why null
would be an object type, I recommend checking out "The history of typeof null" by 2ality. Another interesting behavior is that if you use the typeof
operator twice after each other, you will always get a "string" type.
typeof typeof 1 // Returns "string"
typeof typeof true // Returns "string"
typeof typeof [] // Returns "string"
typeof typeof null // Returns "string"
We can also use the typeof
operator to dynamically assign the type for other variables. This can be done in the following way:
// Assigning type to a variable using the typeof operator
const variable1: string = 'string'
const variable2: typeof variable1 = 42 // This will throw an error: "Type 'number' is not assignable to type 'string'"
Using instanceof
It's also worth noting that if you need to check whether your variable is a type of a class, you need to use the instanceof
keyword instead of the typeof
operator.
class Fruit {}
const fruit = new Fruit()
// This will return true
if (fruit instanceof Fruit) {
// Call method only available on Fruit classes
fruit.grow()
}
Since the fruit
variable was created as a new instance of the Fruit
class, the if statement will be evaluated to true
.
Using the instanceof
operator will always return a boolean value (true
or false
).
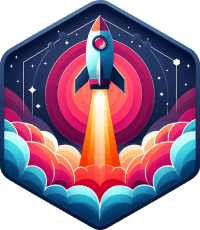
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
