How to Fix "React Hook is Called Conditionally" Errors
The error "React Hook is called conditionally" happens when you try to call a React hook inside a loop, after a condition, or inside a function. To solve the error, make sure you call hooks at the top level. Take a look at the following example:
export default function App() {
if (condition) {
return null
}
const [count, setCount] = useState(0)
return (...)
}
Here we are creating a hook after a condition that will trigger the error. To resolve it, simply move the hook above the condition.
export default function App() {
const [count, setCount] = useState(0)
if (condition) {
return null
}
return (...)
}
The reason hooks must be called at the top level is because they need to be called in the same order each time a component renders, otherwise, React will have problems preserving the state of hooks between multiple render calls.
The same error can also occur when you try to use a hook inside a loop, or a function. Instead, if you want to run an effect conditionally, you can turn things around and define your logic inside the hook.
// π΄ Won't work, hook is called inside a function
export default function App() {
const setCount = () => {
const [count, setCount] = useState(0)
...
}
return (...)
}
// π΄ Won't work, hook is called inside a loop
export default function App() {
data.map((item, index) => {
const [count, setCount] = useState(index)
...
})
return (...)
}
// π΄ Won't work, hook is called conditionally
export default function App() {
if (condition) {
useEffect(() => {
}, [])
}
return (...)
}
// βοΈ Will work, condition is called within the hook
export default function App() {
useEffect(() => {
if (condition) {
}
}, [])
return (...)
}
Also, make sure you only call hooks inside React components, or from other custom hooks, otherwise, you will run into the following error: "React Hook "useState" cannot be called at the top level. React Hooks must be called in a React function component or a custom React Hook function."
// π΄ Won't work, hook is called outside of a component
const [count, setCount] = useState(0)
export default function App() {
return (...)
}
// βοΈ Always call hooks from a component
export default function App() {
const [count, setCount] = useState(0)
return (...)
}
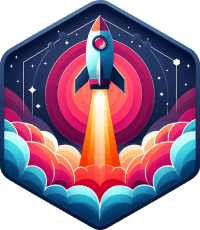
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: