Two Ways to Get Enum Keys by Values in TypeScript
There are two ways to get enum keys by values in TypeScript. You can either use bracket notation or a combination of Object.values
and Object.keys
. Here is an example of using bracket notation:
enum Countries {
Argentina = 'AR' as any,
Armenia = 'AM' as any,
Australia = 'AU' as any,
Austria = 'AT' as any
}
// This will log "Argentina" to the console
console.log(Countries['AR'])
Notice that we typed all of them as any
. It is important to type them, as otherwise, you will get undefined
back. While the above works, it's a quick and dirty solution that can break in certain versions of TypeScript.
The other more reliable way to get enum keys by values is by using a combination of Object.values
with Object.keys
. Take a look at the following example:
enum Countries {
Argentina = 'AR',
Armenia = 'AM',
Australia = 'AU',
Austria = 'AT'
}
const keyIndex = Object.values(Countries).indexOf('AM')
// Returns "Armenia"
console.log(Object.keys(Countries)[keyIndex])
This works by first getting the index of the value with "AM". This can be done by calling Object.values
, and then getting the index of the value with the indexOf
function.
Object.values(Countries)
// This will return the following array (and so keyIndex will be 1):
-> (4) ["AR", "AM", "AU", "AT"]
We can then use this index to grab the proper key by calling Object.keys
, which again returns an array, but instead of returning the object values, it returns the keys that we need.
Object.values(Countries)
// This will return all keys associated with the enum
-> (4) ["Argentina", "Armenia", "Australia"...]
Creating a Helper Function for Getting Enum Keys
We can also create a helper function from the above code example to reuse it later if needed. Take a look at the following code:
const getKey = (obj, value) => {
const keyIndex = Object.values(obj).indexOf(value)
return Object.keys(obj)[keyIndex]
}
getKey(Countries, 'AR') // Returns "Argentina"
getKey(Countries, 'AM') // Returns "Armenia"
getKey(Countries, 'AU') // Returns "Australia"
getKey(Countries, 'AT') // Returns "Austria"
This function expects an enum (or any object for that matter) and a value that you want to use to get the key. In fact, we can also use the same function with any other object to get the key as a string.
// We can also use the function on plain JavaScript objects too
const countries = {
Argentina: 'AR',
Armenia: 'AM',
Australia: 'AU',
Austria: 'AT'
}
// Returns "Australia"
getKey(countries, 'AU')
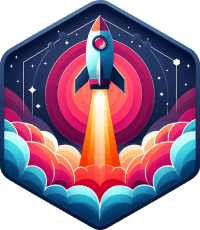
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
