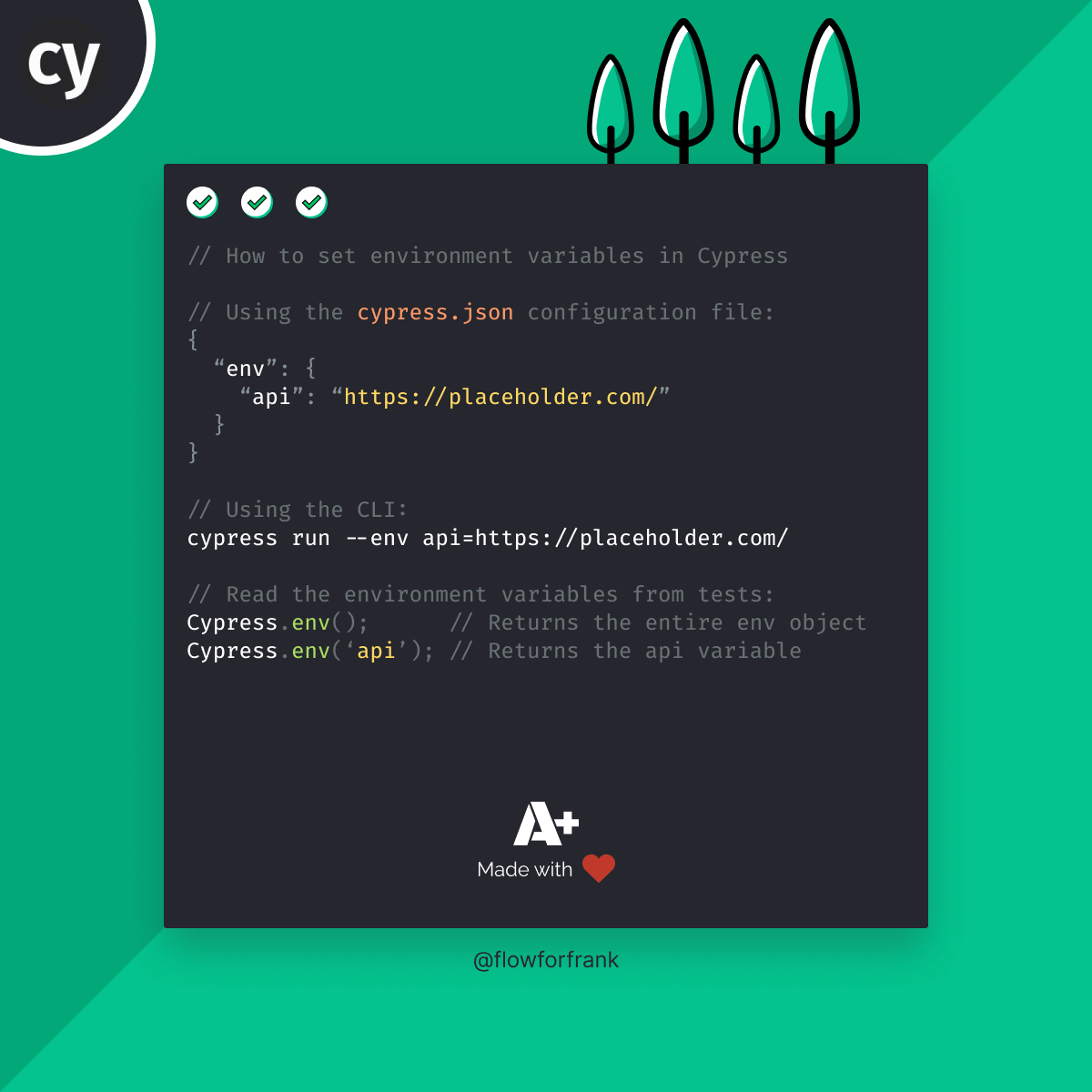
How to Set Environment Variables in Cypress
You can set environment variables in Cypress by using your cypress.json
configuration file, your CLI, plugins, or test configuration.
Using the Configuration File
The most common approach is to use your cypress.json
configuration file to add environment variables, which you can do so using the env
property:
{
"env": {
"api": "https://placeholder.com/"
}
}
Using the CLI
You can also pass environment variables to Cypress using the CLI by passing the --env
flag:
cypress run --env api=https://placeholder.com/
This can be especially useful if you need to define multiple commands for Cypress that uses slightly different configurations. For example, you may want to have different commands for a staging or prod env:
"scripts": {
"cypress:staging": "cypress run --env api=staging",
"cypress:prod": "cypress run --env api=prod",
},

Using Plugins
Plugins can also be used to programmatically change add, remove, or modify your environment variables in your config:
module.exports = (on, config) => {
console.log('Your Cypress config is', config);
config.env.NODE_ENV = 'development';
return config;
};
In case you want to define environment variables based on some conditions, then you can easily implement that here.
Using Test Configuration
Lastly, you can also define environment variables right inside your test files on a case by case basis, by passing an env
object to your describe
or it
blocks:
const config = {
env: {
api: 'https://placeholder.com/'
}
};
describe('env variables', config, () => { ... });
it('Should set the right env variables', config, () => { ... });
Apart from the above-mentioned solution, Cypress also lets you create cypress.env.json
files or export OS-level environment variables with variable names starting with CYPRESS_*
or cypress_*
.
Using Environment Variables in Tests
So how do you actually use these environment variables in your test cases? You can access them by using Cypress.env
:
Cypress.env(); // Returns the entire env object
Cypress.env('api'); // Returns the api variable
Want to learn Cypress from end to end? Check out my Cypress course on Educative where I cover everything:

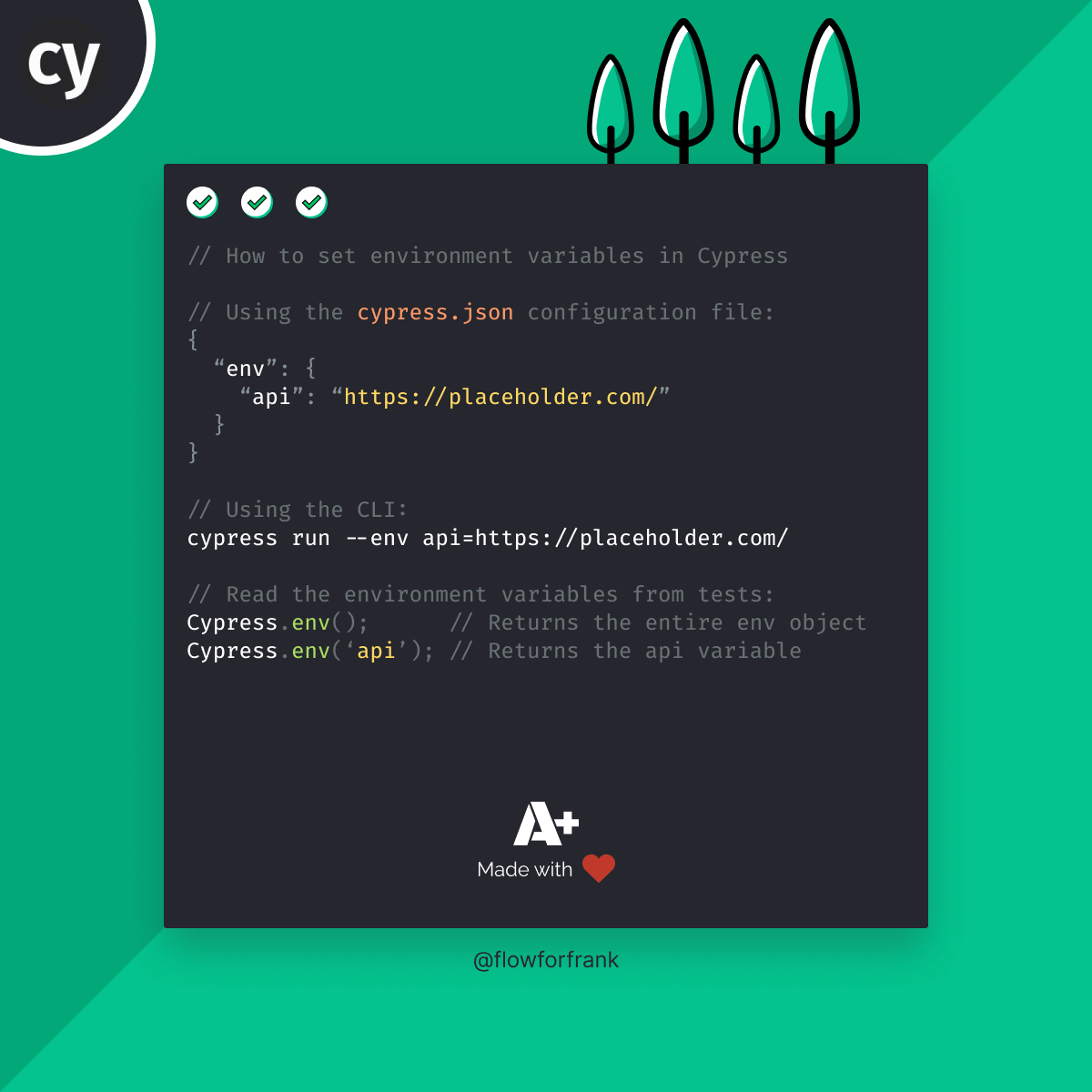
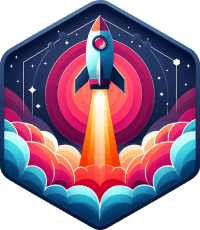
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: