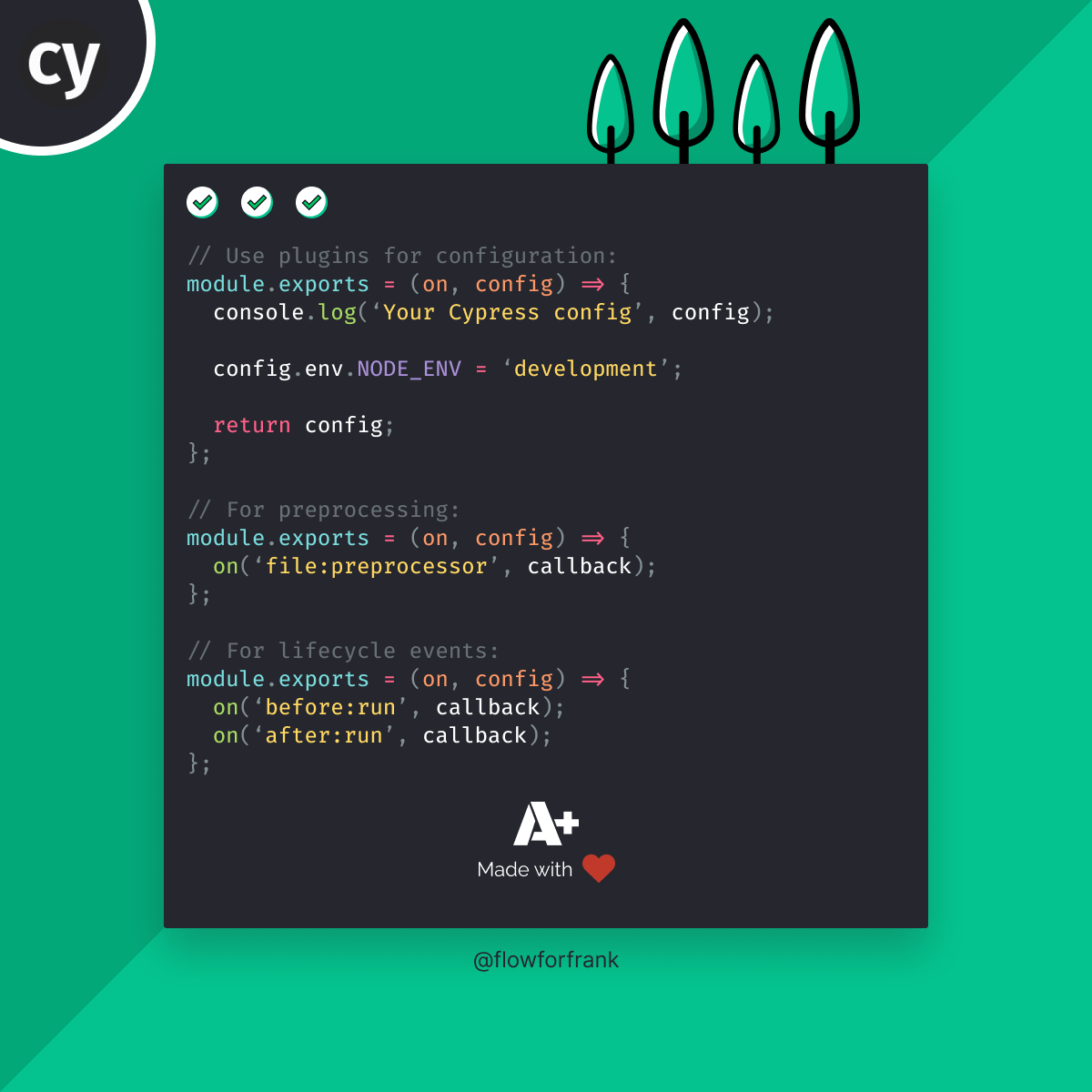
How to Use Plugins in Cypress
Plugins in Cypress can be used for a number of different things, such as configuration, preprocessing, hooking into lifecycle events, or defining custom tasks. Let's go quickly over them one by one.
Configuring Cypress
Most commonly, you will see plugins used for configuring the environment for your test suite. For example, adding environment variables to your configuration object programmatically in the following way:
module.exports = (on, config) => {
console.log('Your Cypress config is', config);
config.env.NODE_ENV = 'development';
return config;
};
Here you have access to the entire configuration with the config
variable. Note that Cypress expects a function to be exported from this file.
Make sure you always return your config
object at the end of the function call.
Preprocessing
Plugins can also be used for preprocessing. You may have also noticed that there is an on
argument in the exported function. This can be used exactly for that.
For example, if you are working with TypeScript, this is where you can preprocess and transpile your TypeScript files, customize your compile or Babel settings, or add support for ES features. To hook into the preprocess event, add the following to your plugin file:
module.exports = (on, config) => {
on('file:preprocessor', callback);
};

Hooking into Lifecycle Events
Apart from the file:preprocessor
event, you can also hook into other lifecycle events to further customize the behavior of Cypress. These are events that can help you run code before or after your test suite or a single spec file. Let's see how:
module.exports = (on, config) => {
// Run before and after your test suite
on('before:run', callback);
on('after:run', callback);
// Run before and after a single spec file
on('before:spec', callback);
on('after:spec', callback);
};
Defining Tasks
Last but not least, you can also use plugins to define custom tasks that you can reuse throughout your spec files. This can be done using the on('task')
call. Let's see an example. Say you have the following custom task defined in your plugins file:
module.exports = (on, config) => {
on('task', {
log(message) {
console.log(message);
return null;
}
});
};
You would call this task in your spec file using the built-in cy.task
command, passing in the name of the function:
it('Should call the above defined task', () => {
cy.task('log');
});
You can also install existing plugins for Cypress. You can find a link to the full list of available plugins in the resources section below.
Want to learn Cypress from end to end? Check out my Cypress course on Educative where I cover everything:

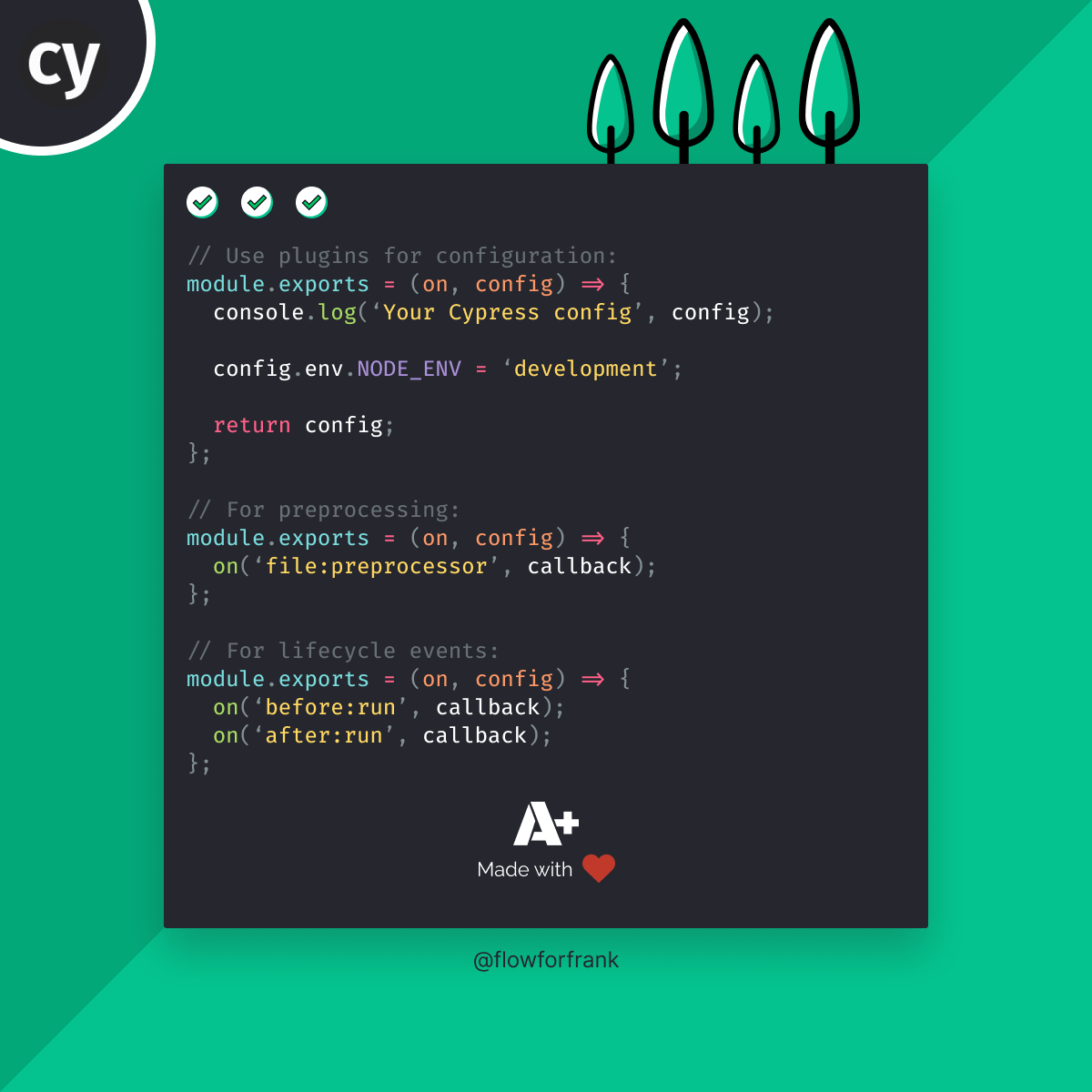
Resources:
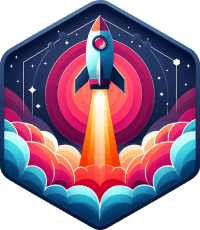
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: