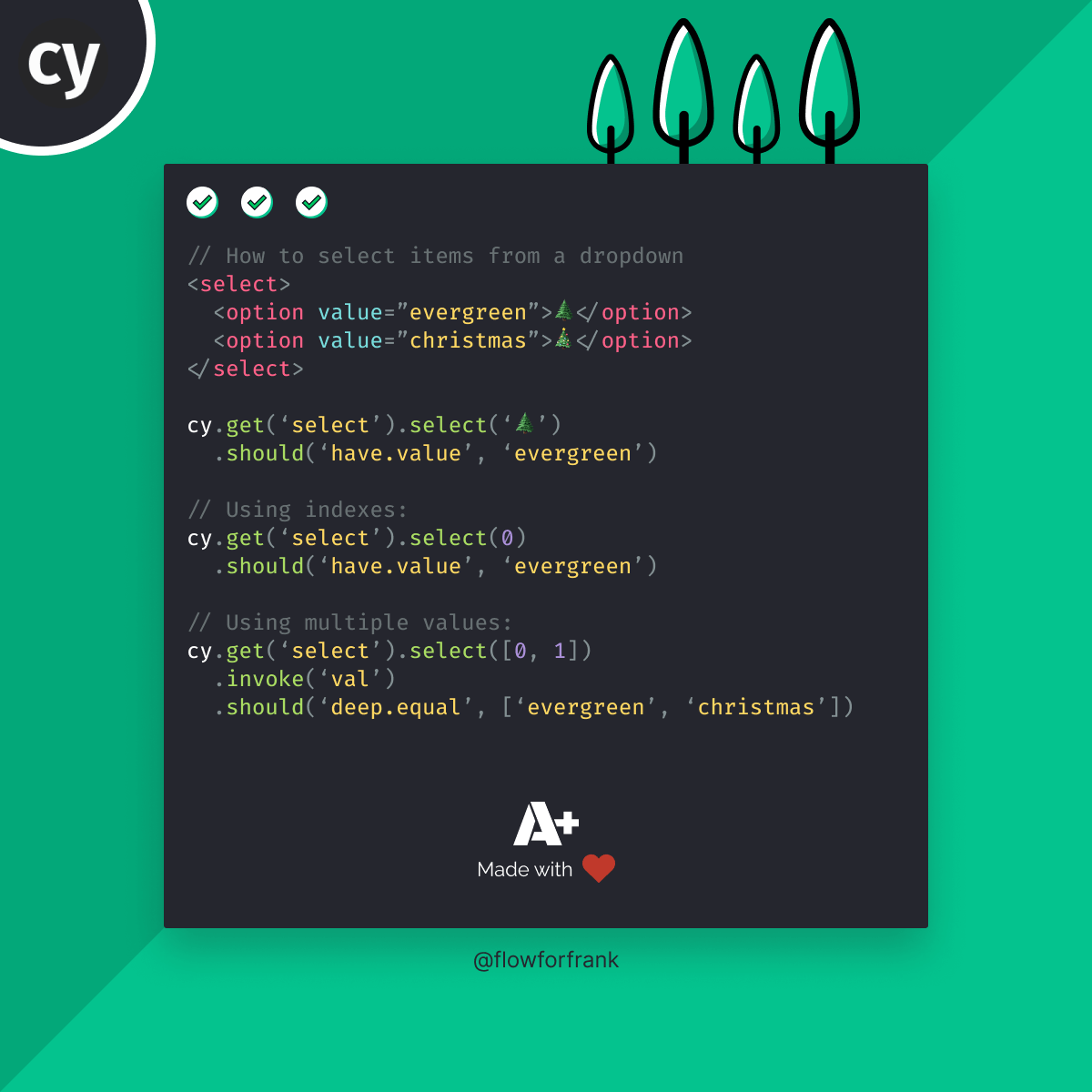
How to Select Multiple Items From a Dropdown in Cypress
If you need to select an item from a dropdown in Cypress, you can use the select
command, passing the value, text content, or the index of the option you want to select. Let's say you have the following select
in your document:
<select>
<option value="evergreen">π²</option>
<option value="christmas">π</option>
</select>
In order to select the first option in Cypress, we can do one of the following:
cy.get('select').select('π²').should('have.value', 'evergreen')
cy.get('select').select(0).should('have.value', 'evergreen')
cy.get('select').select('evergreen').should('have.value', 'evergreen')
Apart from passing the text content, we can pass an index to the select
command, starting from 0. We also have the possibility to use the value
attribute to grab the necessary option
. The three lines in the above code example are equivalent. All of them select the first option
from the select
.
How to Grab Multiple Items from a Dropdown
To grab more than one item from a dropdown, we can pass an array of values, text content, or indexes.
// Using text content
cy.get('select').select(['π²', 'π'])
.invoke('val')
.should('deep.equal', ['evergreen', 'christmas'])
// Using indexes
cy.get('select').select([0, 1])
.invoke('val')
.should('deep.equal', ['evergreen', 'christmas'])
// Using values
cy.get('select').select(['evergreen', 'christmas'])
.invoke('val')
.should('deep.equal', ['evergreen', 'christmas'])
Then we can use a combination of invoke('val')
with should('deep.equal')
to verify all of their values in one go.
Select Items from a Disabled Dropdown
In case you are working with a disabled dropdown, but you still need to interact with it to verify some cases, you can pass a force: true
configuration to your select
command:
cy.get('select').select('π²', { force: true })
.should('have.value', 'evergreen')
This will work regardless of whether your select
is not displayed, or it's just disabled.
Want to learn Cypress from end to end? Check out my Cypress course on Educative where I cover everything:

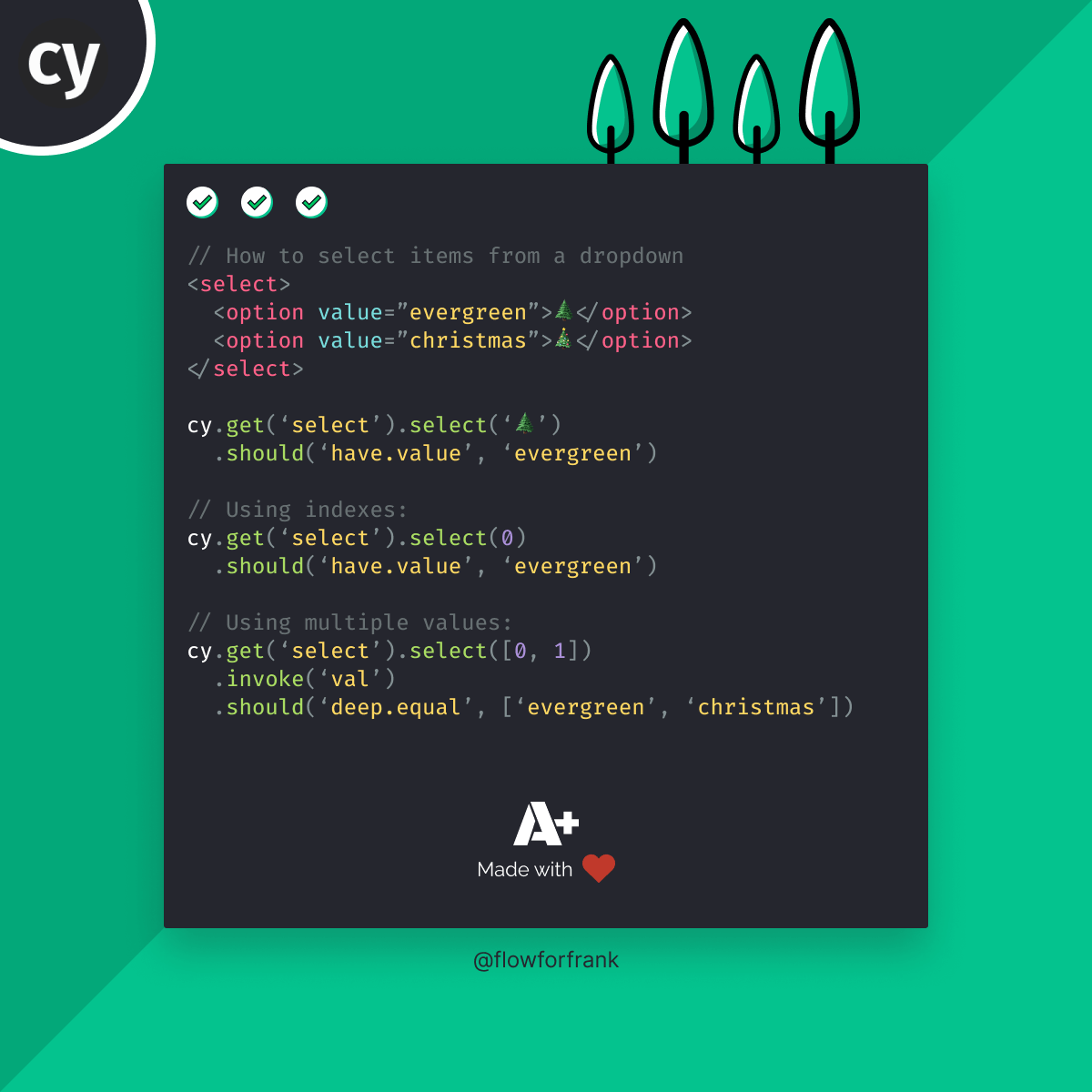

Resources:
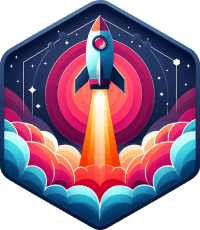
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: