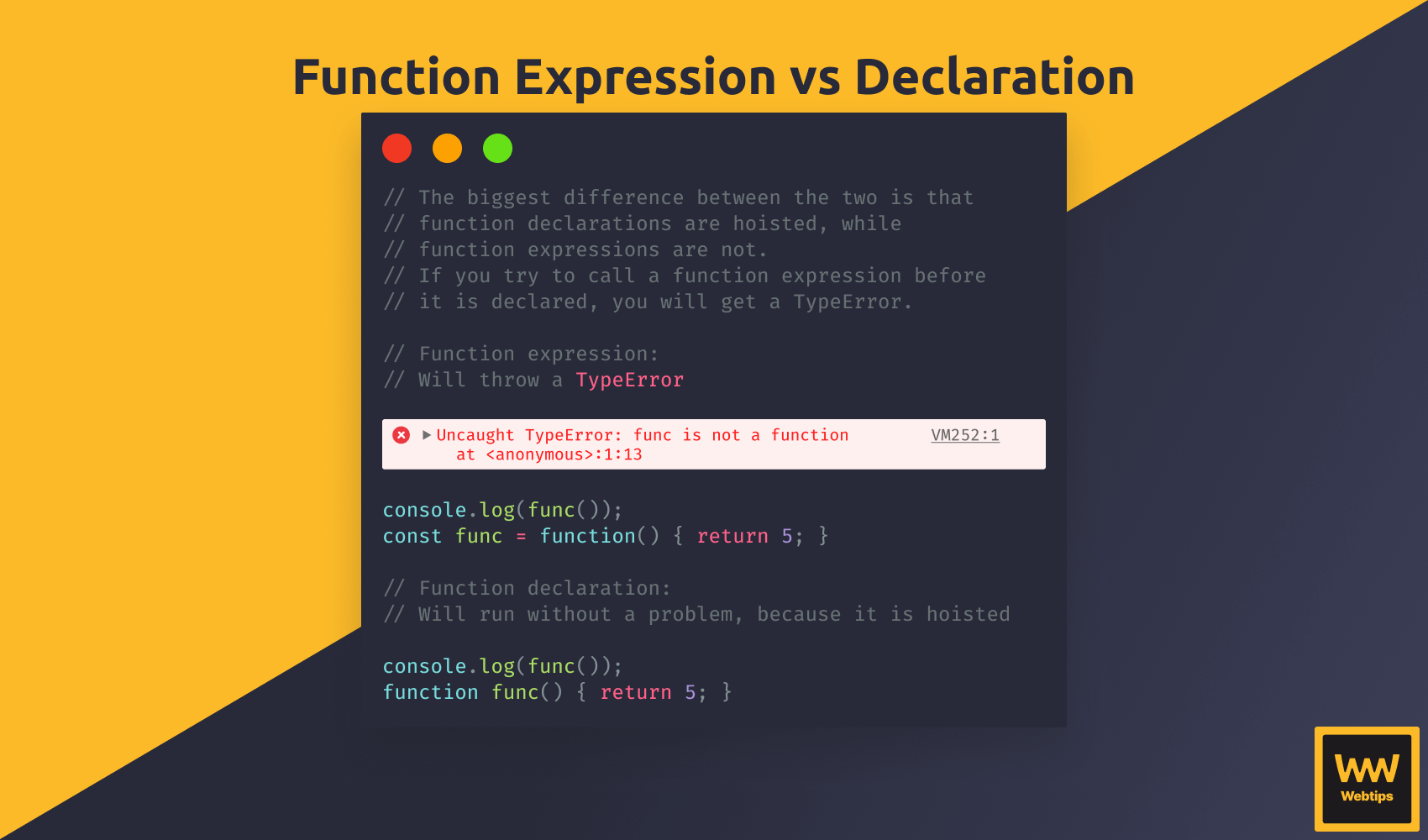
The Difference Between Function Expression and Function Declaration in JavaScript
Function expressions and declarations have two major differences. One is that for function expressions, you are assigning the function to a variable. In a function declaration, you don't:
// Function expression
// Will throw a TypeError
console.log(func());
var func = function() { return 5; }
// Function declaration
// Will run without a problem, because it is hoisted
console.log(func());
function func() { return 5; }
The biggest difference however is that expressions are not hoisted. If you call the function before it was initialized, you will get a type error:

Whichever you choose to use throughout your code, make sure you stay consistent to avoid unexpected behaviors.
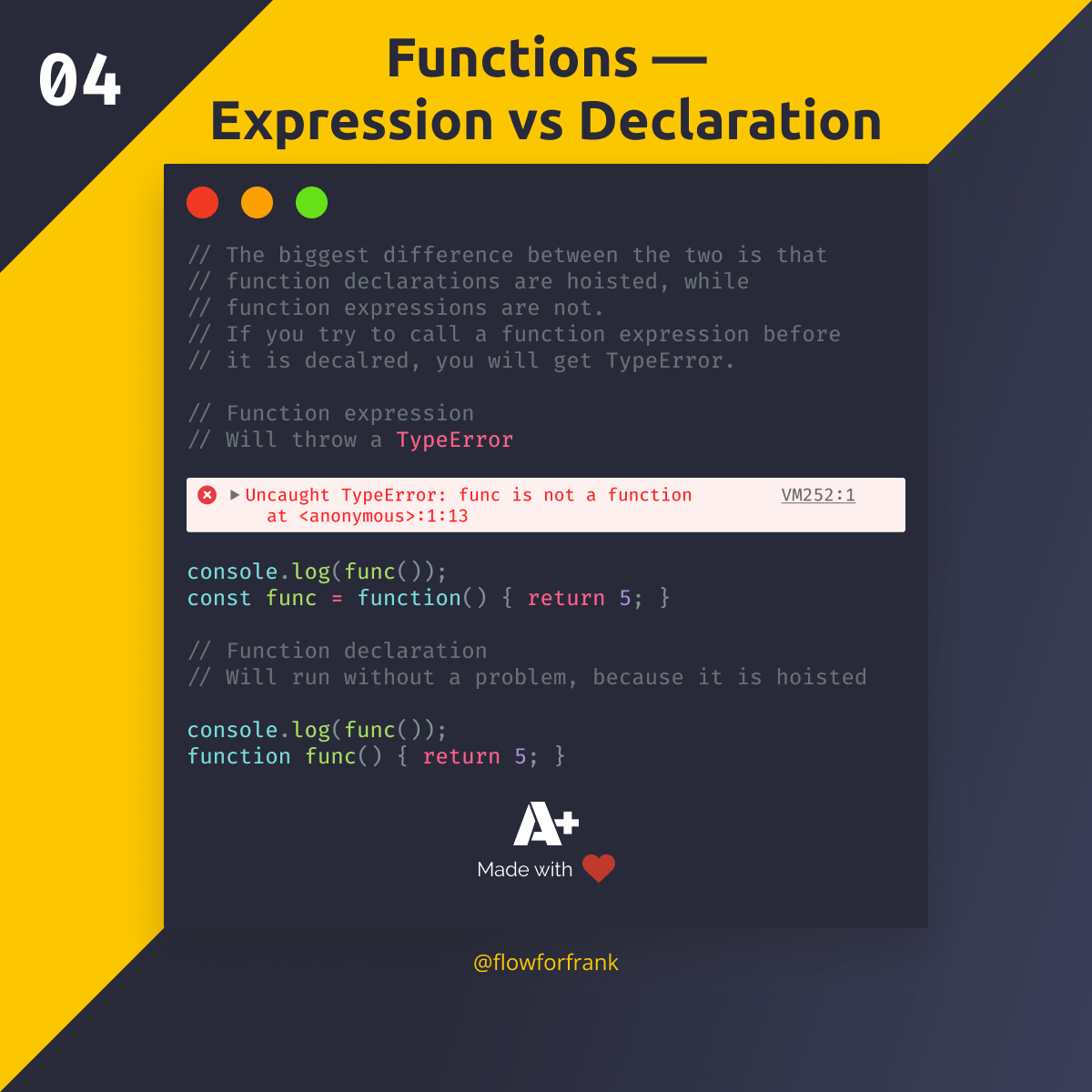
Resources:
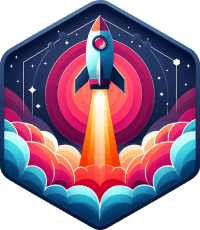
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: