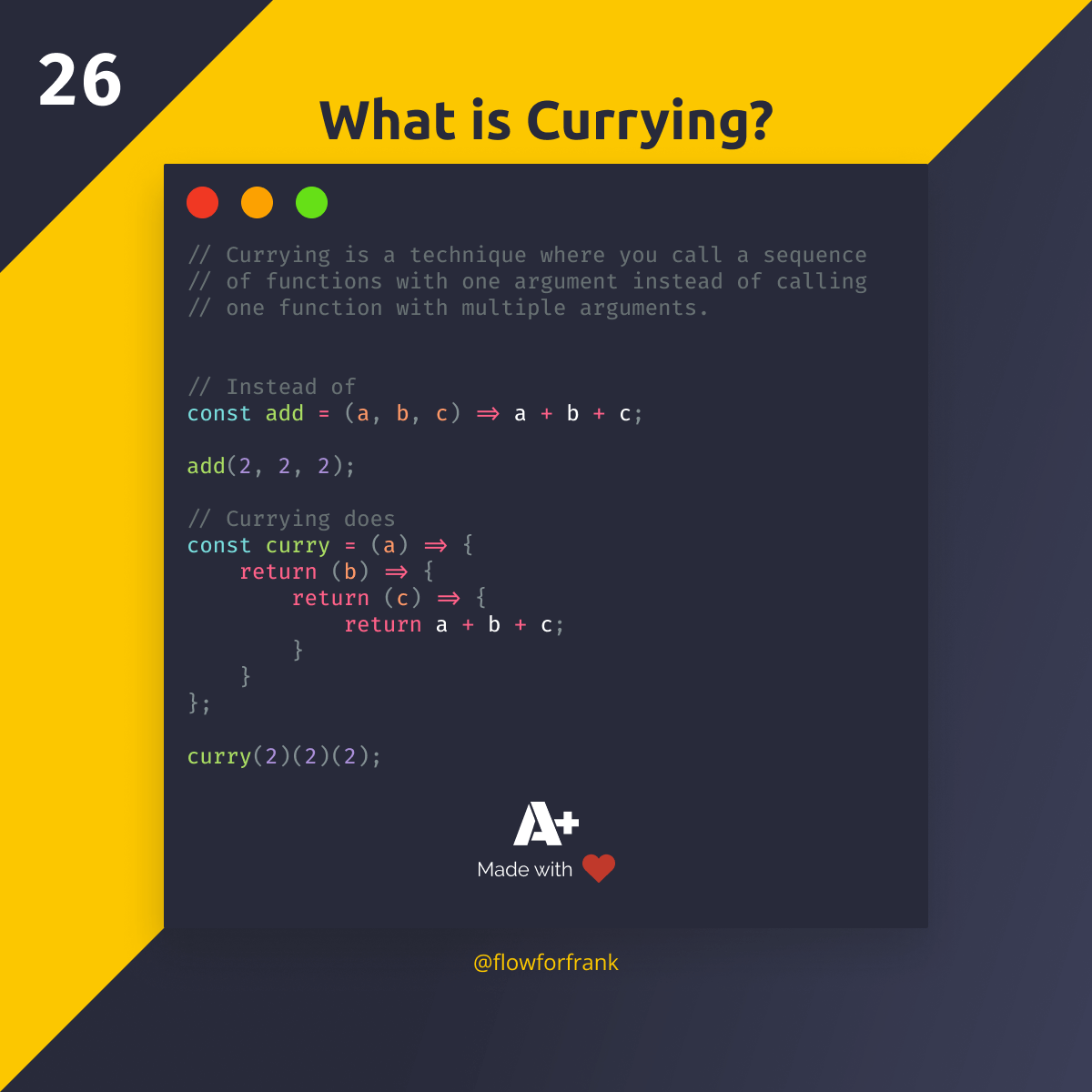
What is Currying in JavaScript?
Currying is a technique where you call a sequence of functions with one argument instead of calling one function with multiple arguments. You call a function with the first argument which returns a function, which you call with the second argument and so on:
// Instead of
const add = (a, b, c) => a + b + c;
add(2, 2, 2);
// Currying does
const curry = (a) => {
return (b) => {
return (c) => {
return a + b + c;
}
}
};
curry(2)(2)(2);
And why is it called "currying"? According to Wikipedia, the term has been coined by Christopher Strachey back in 1967 as a reference to Haskell Curry who was a mathematician and logician in the 20th century.
An alternative name has also been proposed called "Schönfinkelisation", as a reference to Moses Schönfinkel, a Russian mathematician and logician.
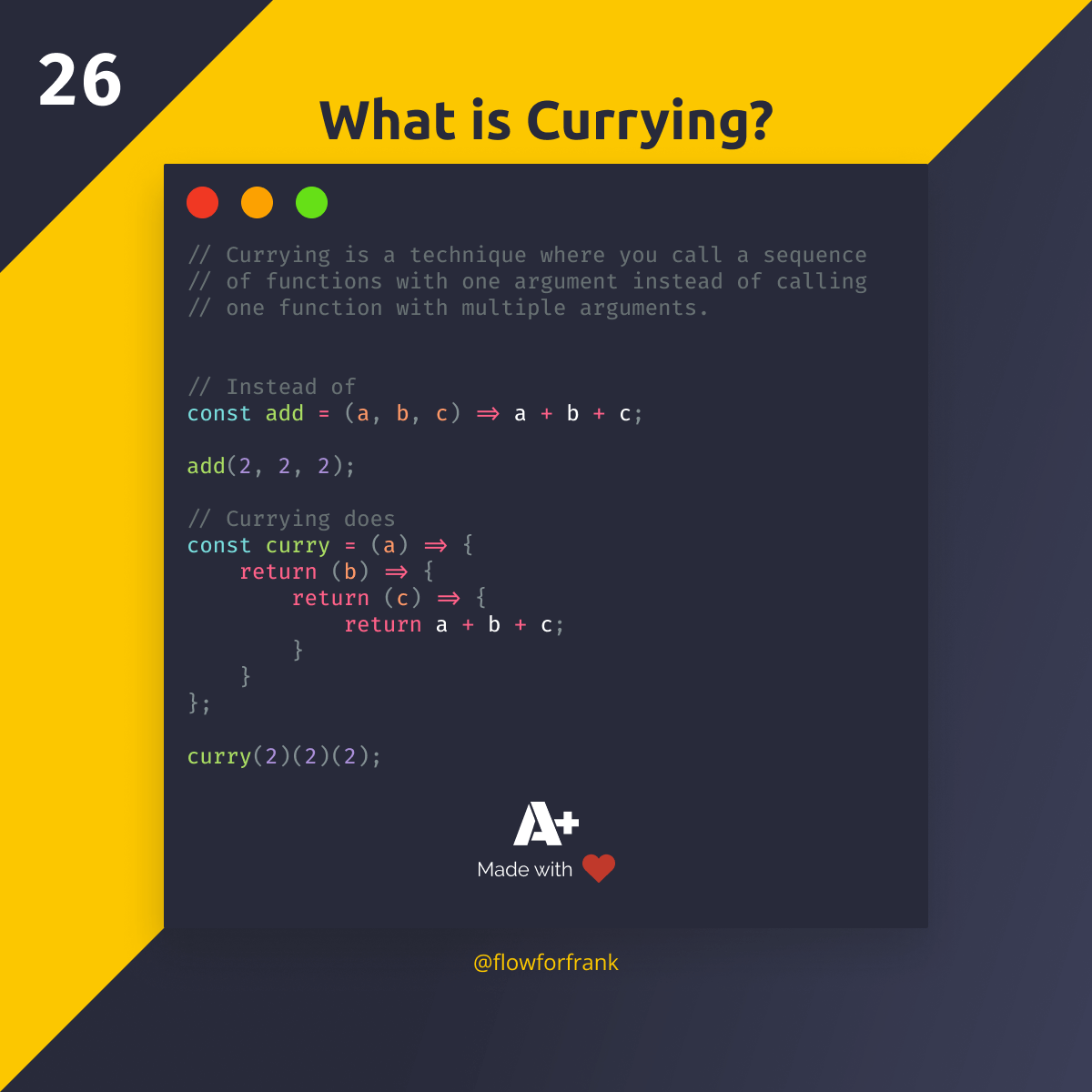
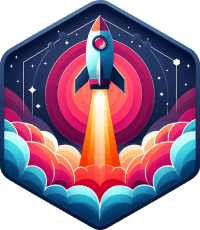
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: