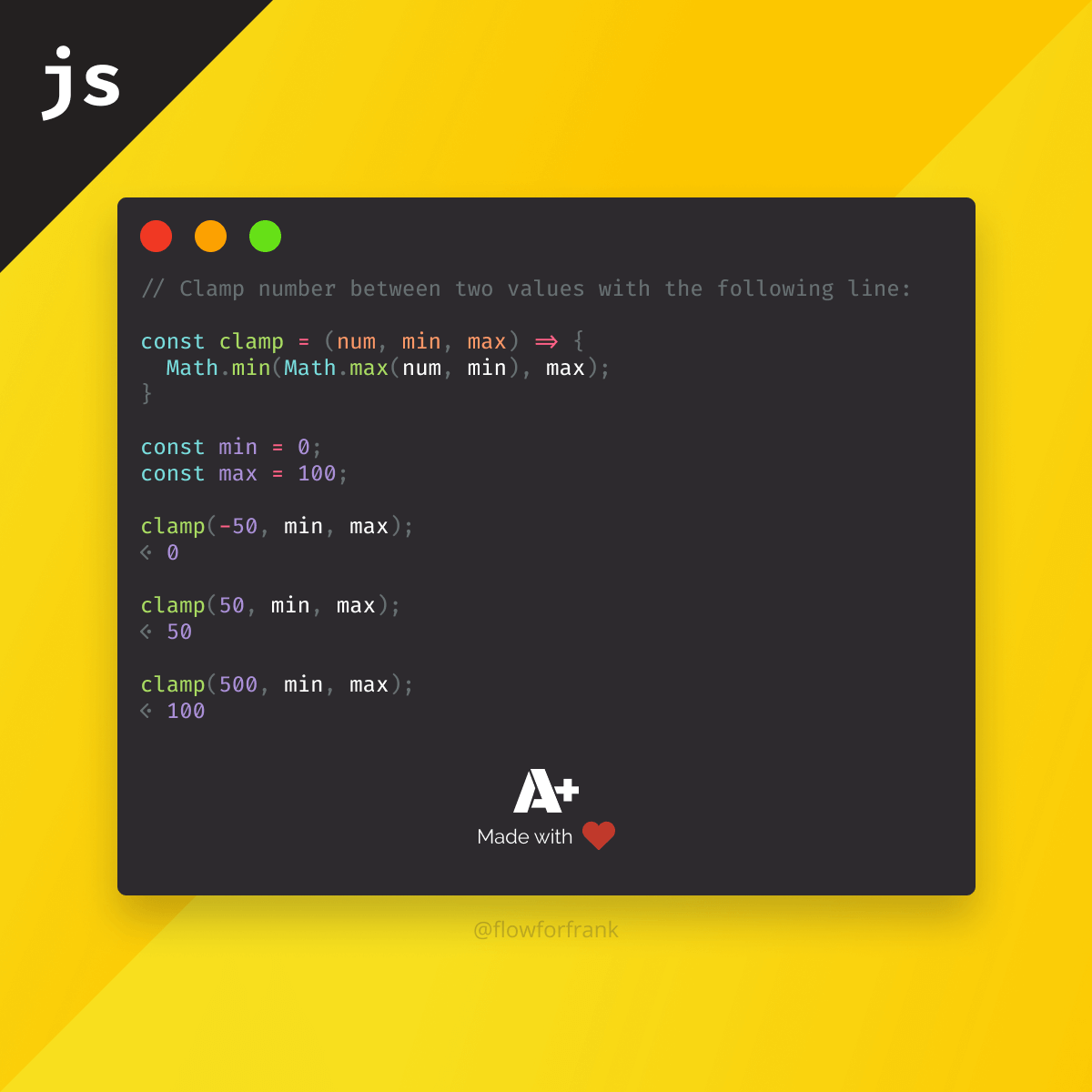
How to Clamp Numbers in JavaScript
Use the following function in JavaScript to easily clamp numbers between a min and a max value:
const min = 0
const max = 100
// Clamp number between two values with the following line:
const clamp = (num, min, max) => Math.min(Math.max(num, min), max)
clamp(-50, min, max) // Will return: 0
clamp(50, min, max) // Will return: 50
clamp(500, min, max) // Will return: 100
Explanation
Let's break this code down to fully understand what is going on. We start with a number, and min and max values. Let's say the number we want to cap is 50, our min is 0, and our max is 100. We pass our number to Math.max
, along with our min value:
Math.max(50, 0) // -> 50
This will return the higher number from the two, which means we get 50 back. Next, we pass this to Math.min
along with our max value:
Math.min(50, 100) // -> 50
This time, this will return the lowest number from the passed arguments. Hence:
- If value < min, it will return the minimum allowed number
- If value > max, it will return the maximum allowed number
- If value > min and value < max, it will return the passed number
Live Example
You can test the functionality in the following interactive widget with your desired values to check if the function suits your needs.

Official Support
Using the same function, we can make it global by extending the Number
object in the following way:
Number.prototype.clamp = (num, min, max) => Math.min(Math.max(num, min), max)
(-50).clamp(-50, min, max)
(50).clamp(50, min, max)
(500).clamp(500, min, max)
However, keep in mind this is considered to be a bad practice and should be avoided. Only use it if you need compatibility with newer features.
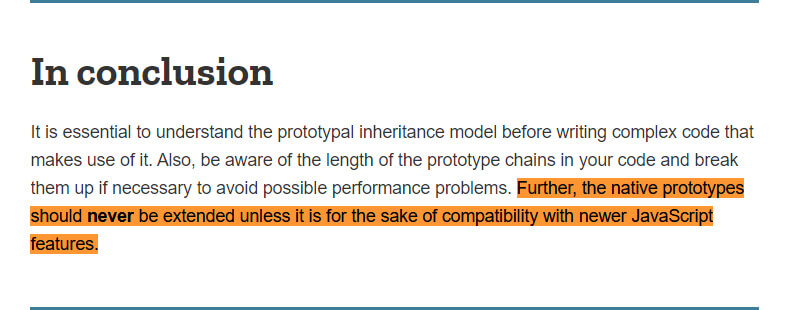
Instead, if you use a modern framework, you can simply include it at the root of your project or store it in a global namespaced object (eg.: app.utils.clamp
). There is also a proposal for a built-in Math.clamp
function, however, it's still in draft. Until it gets widely adopted, you need to polyfill it.
Resources
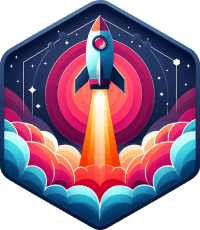
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: