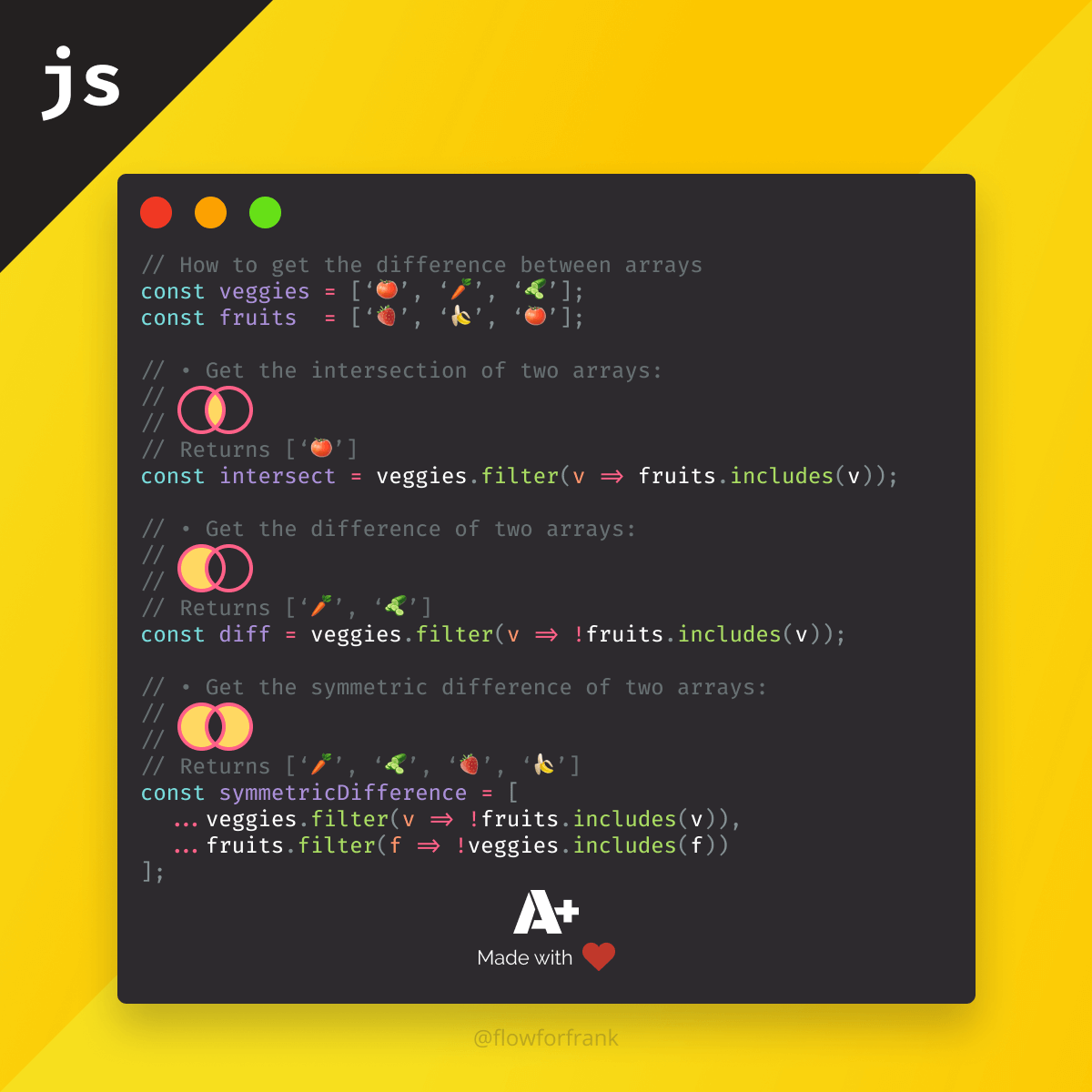
How to Get Intersection of Two Arrays in JavaScript
To get the difference of two arrays in JavaScript, you can use the combination of filter
and includes
. Imagine you have the following two arrays and you want to find out which elements are included in both:
const veggies = ['π
', 'π₯', 'π₯'];
const fruits = ['π', 'π', 'π
'];
To get their intersection, you can use the following call:
const intersection = veggies.filter(v => fruits.includes(v));
To get their differencem eg.: only the ones that veggies
include, you can do the following:
const difference = veggies.filter(v => !fruits.includes(v));
const difference = fruits.filter(f => !veggies.includes(f));
And in order to get the symmetric difference of two arrays β meaning leave out the ones that are in both arrays β you need to filter both of them and either use concat
or destructuring:
const symmetricDifference = [
...veggies.filter(v => !fruits.includes(v)),
...fruits.filter(f => !veggies.includes(f))
];
Reusable functions
If you need to rely on the same logic multiple times in your codebase, you can reuse them with the following function calls:
const intersect = (a, b) => a.filter(a => b.includes(a));
const difference = (a, b) => a.filter(a => !b.includes(a));
const symmetric = (a, b) => [
...a.filter(a => !b.includes(a)),
...b.filter(b => !a.includes(b))
];
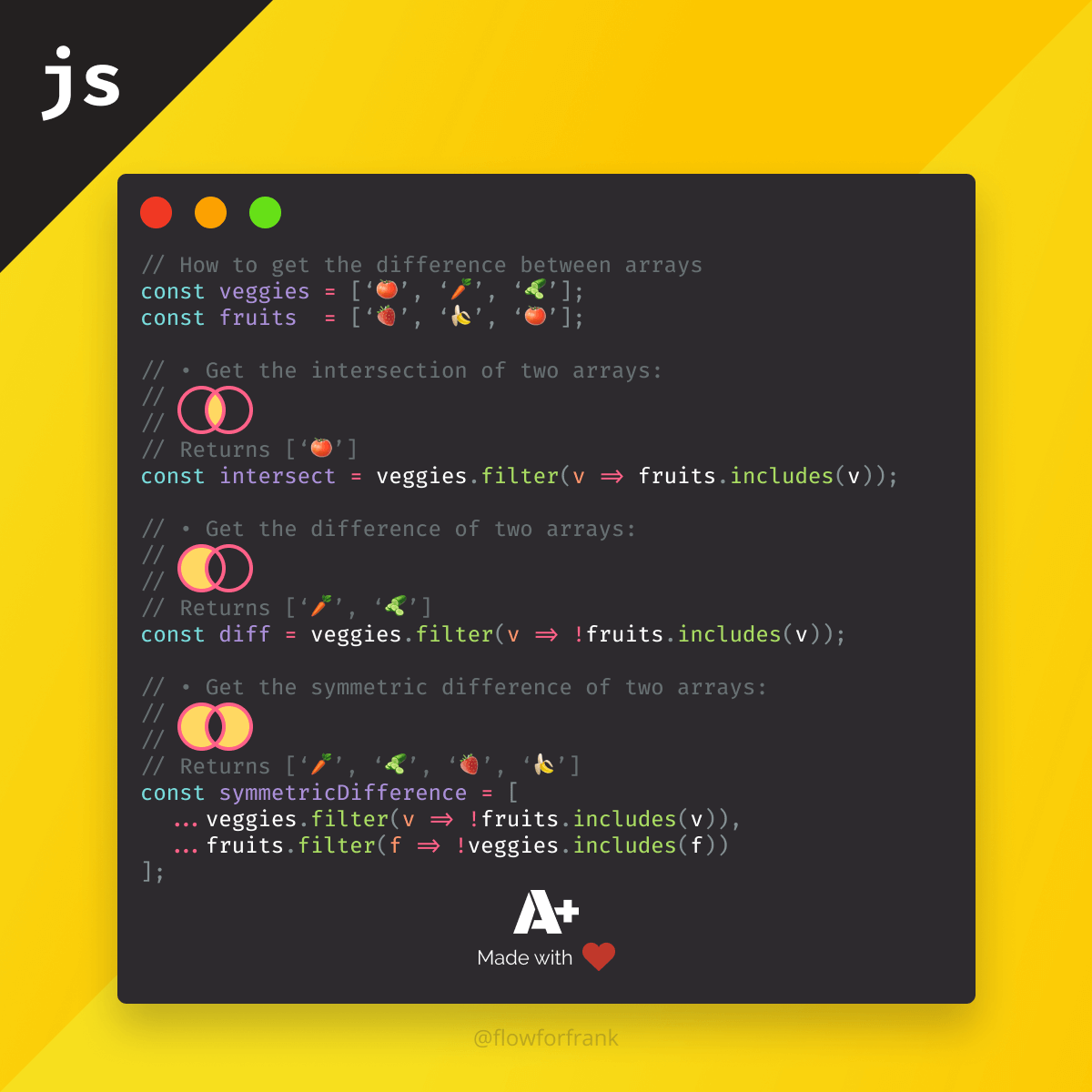
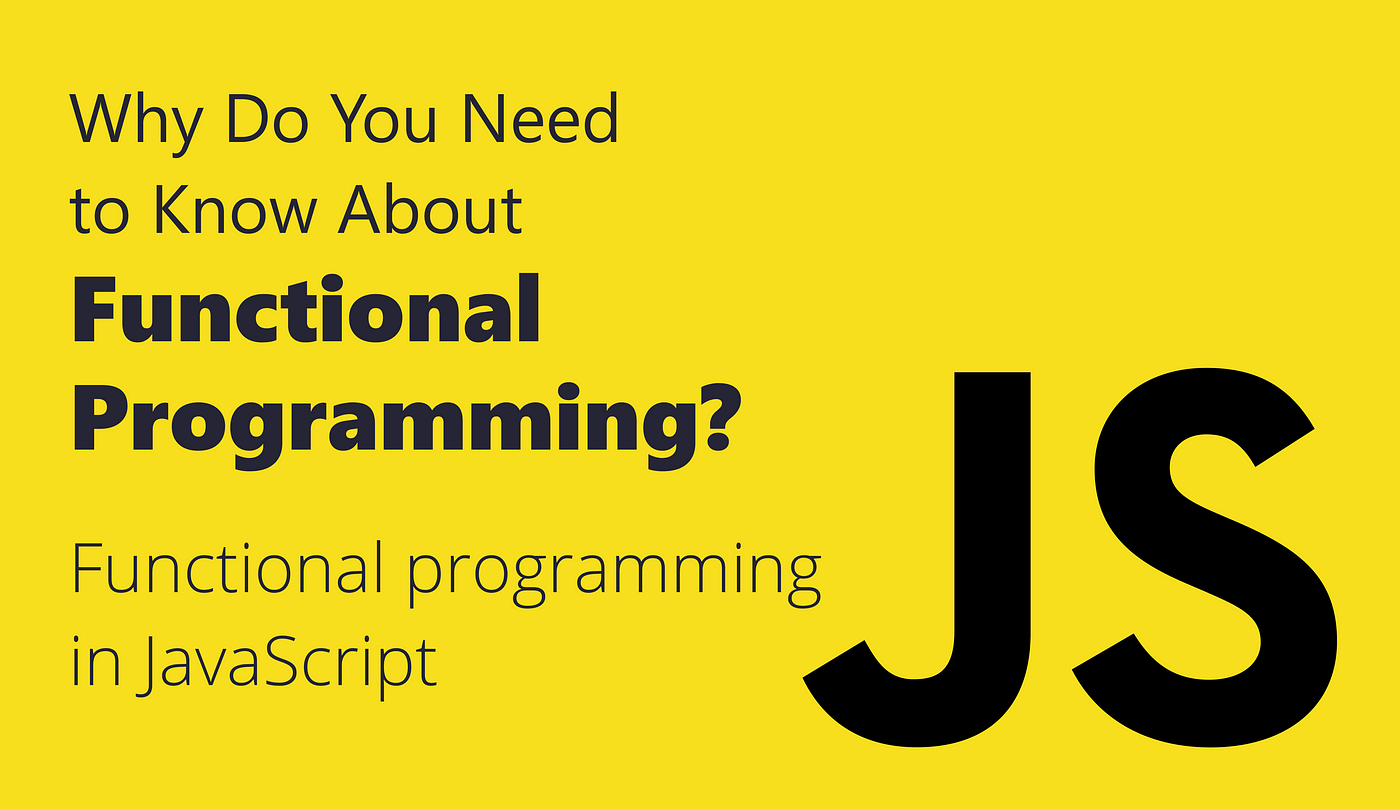
Resources:
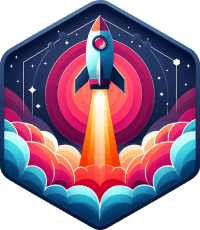
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: