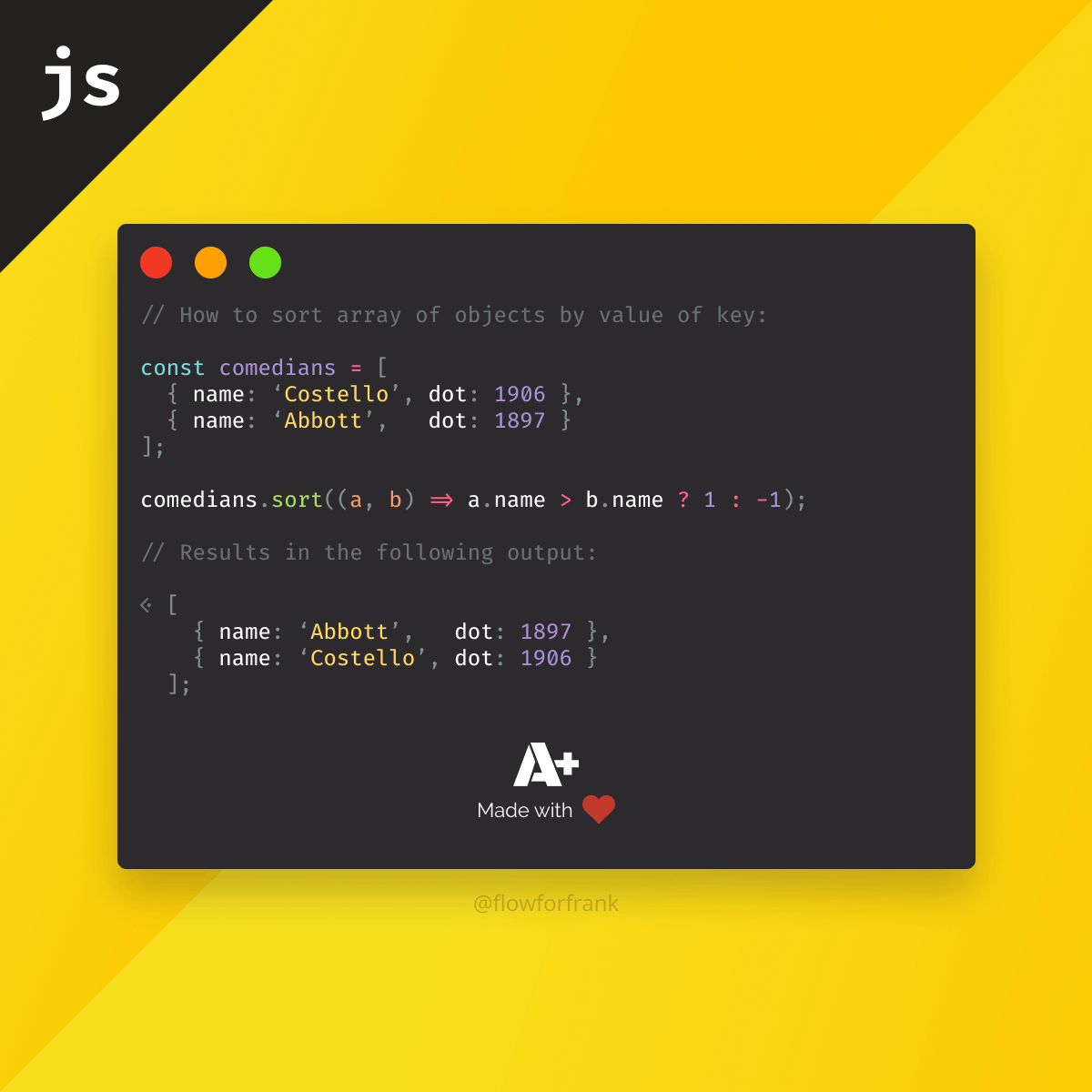
How to Sort Array of Objects in JavaScript
You can easily sort an array of objects in JavaScript based on the value of a given key with the following one-liner:
// How to sort array of objects by value of key:
const comedians = [
{ name: 'Costello', dot: 1906 },
{ name: 'Abbott', dot: 1897 }
];
comedians.sort((a, b) => a.name > b.name ? 1 : -1);
// Results in the following output:
[
{ name: 'Abbott', dot: 1897 },
{ name: 'Costello', dot: 1906 }
];
Based on this solution, you can also create a function to dynamically sort an array of objects, based on the passed key:
const comedians = [
{ name: 'Costello', dot: 1906 },
{ name: 'Abbott', dot: 1897 }
];
const sort = (array, key) => array.sort((a, b) => a[key] > b[key] ? 1 : -1);
sort(comedians, 'name') // Returns the same sorted array as in the previous example
To further enhance this example, you can add the order as the third parameter:
const sort = (array, key, order) => {
const returnValue = order === 'desc' ? -1 : 1;
return array.sort((a, b) => a[key] > b[key] ? returnValue * -1 : returnValue);
};
sort(comedians, 'name', 'desc');
sort(comedians, 'name', 'asc');
Also, if you only need to sort simple data structures rather than objects, you can simply use sort:
[5, 4, 3, 2, 1, 0].sort(); // Will return [0, 1, 2, 3, 4, 5];
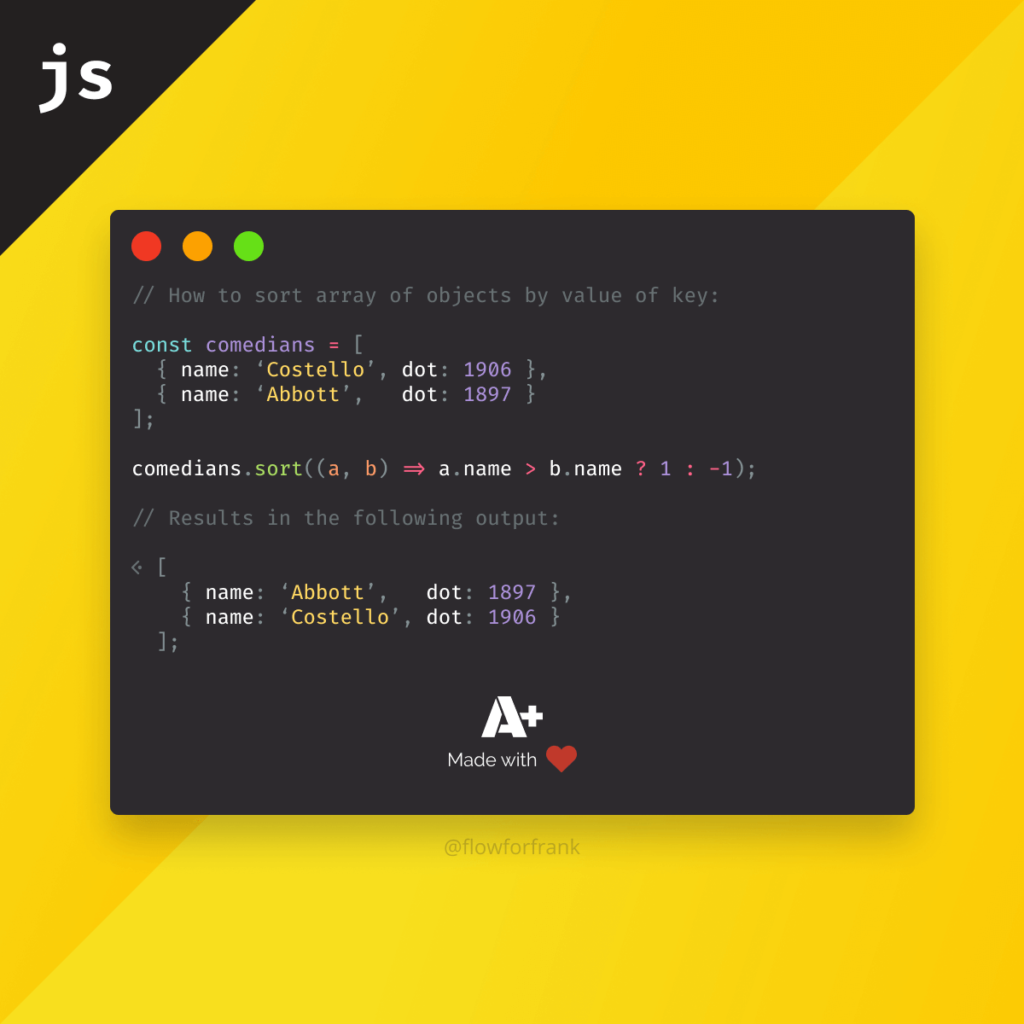
Resource
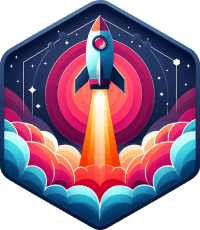
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: