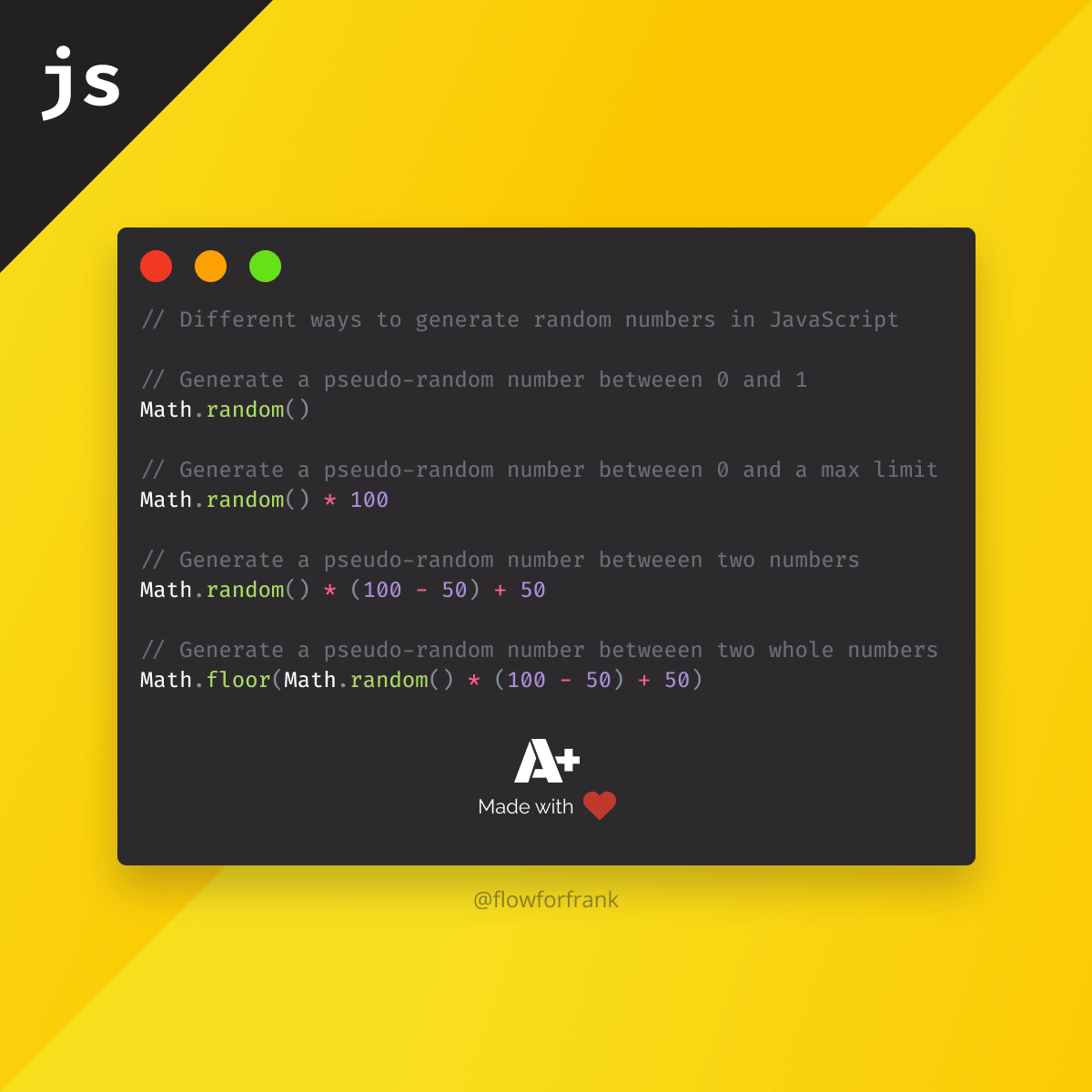
Multiple Random Number Generators for JavaScript
In JavaScript, the Math.random
method can be used in various ways to generate pseudo-random numbers.
A pseudo-random number is a number that statistically appears to be random, but can be produced by a deterministic process.
On its own, the method will return a random number between 0 and 1. This is the simplest way to generate a random number:
// Built-in Random Number Generator
Math.random()
However, we often look for numbers to be generated between two values. By multiplying this with a number of our choice, we can define an upper limit.
Generate With a Max Limit
Math.random() * 10
This way we can say that we want a random number to be generated between 0 and 10. However, we can also define both an upper and a lower limit by introducing yet another number.
Generate Between Two Numbers
// Generate a random number between 5 and 10
Math.random() * (10 - 5) + 5
// The above can be defined using the following formula
Math.random() * (max - min) + min
First, we need to pass a max value minus the min, and then multiply it with Math.random
, then add the min value to the result to make sure it is within the range. We can take this expression one step further.

Generate Between Whole Numbers
If you want to generate random whole numbers β which is more often the case β we can wrap the previous example into Math.floor
to make it work:
Math.floor(Math.random() * (10 - 5) + 5)
Generate X Random Numbers
Lastly, you can also use the below helper function to generate x amount of random numbers using a range:
const generateRandomNumbers = (min, max, times) => {
const randoms = []
for (let i = 0; i < times; i++) {
randoms.push(Math.floor(Math.random() * (max - min) + min))
}
return randoms
}
// Later call it like so
generateRandomNumbers(10, 20, 5)
> [12, 17, 16, 14, 11]
The function expects a min and a max value, and a number defining the number of times we want to generate random numbers. It will return them inside an array. To create the list of random numbers, we can utilize a simple for
loop.
Bonus: Random Letter Generator
Want to also generate random letters? Check out this code example from a previous tutorial that can be used to generate random letters for example to be used in a password:
const generatePassword = passwordLength => {
const defaultCharacters = 'abcdefghijklmnopqrstuvwxyz'
const characters = {
uppercase: defaultCharacters.toUpperCase(),
numbers: '0123456789',
symbols: '~!@-#$'
}
const characterList = [
defaultCharacters,
characters.uppercase,
characters.numbers,
characters.symbols
].join('')
return Array.from({ length: passwordLength }, () => Math.floor(Math.random() * characterList.length))
.map(number => characterList[number])
.join('')
}
// The function expects a length to be passed
generatePassword(10)
> 'PkBAp64lVn'
You can find out more about the implementation details and how this function works from the below tutorial. Thank you for reading through, happy coding!
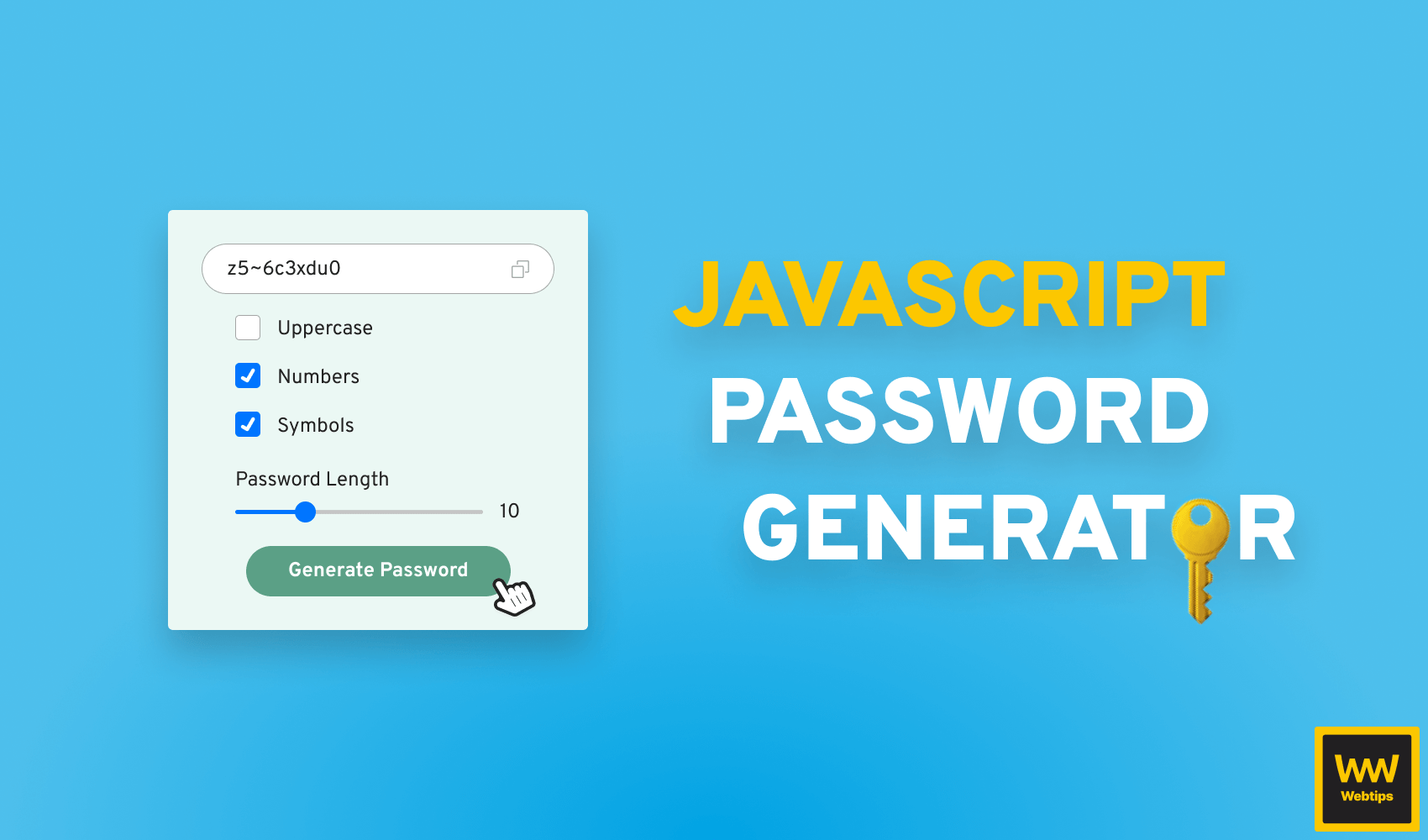
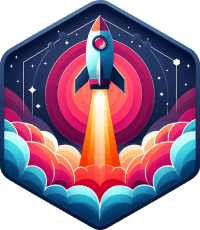
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses
Recommended
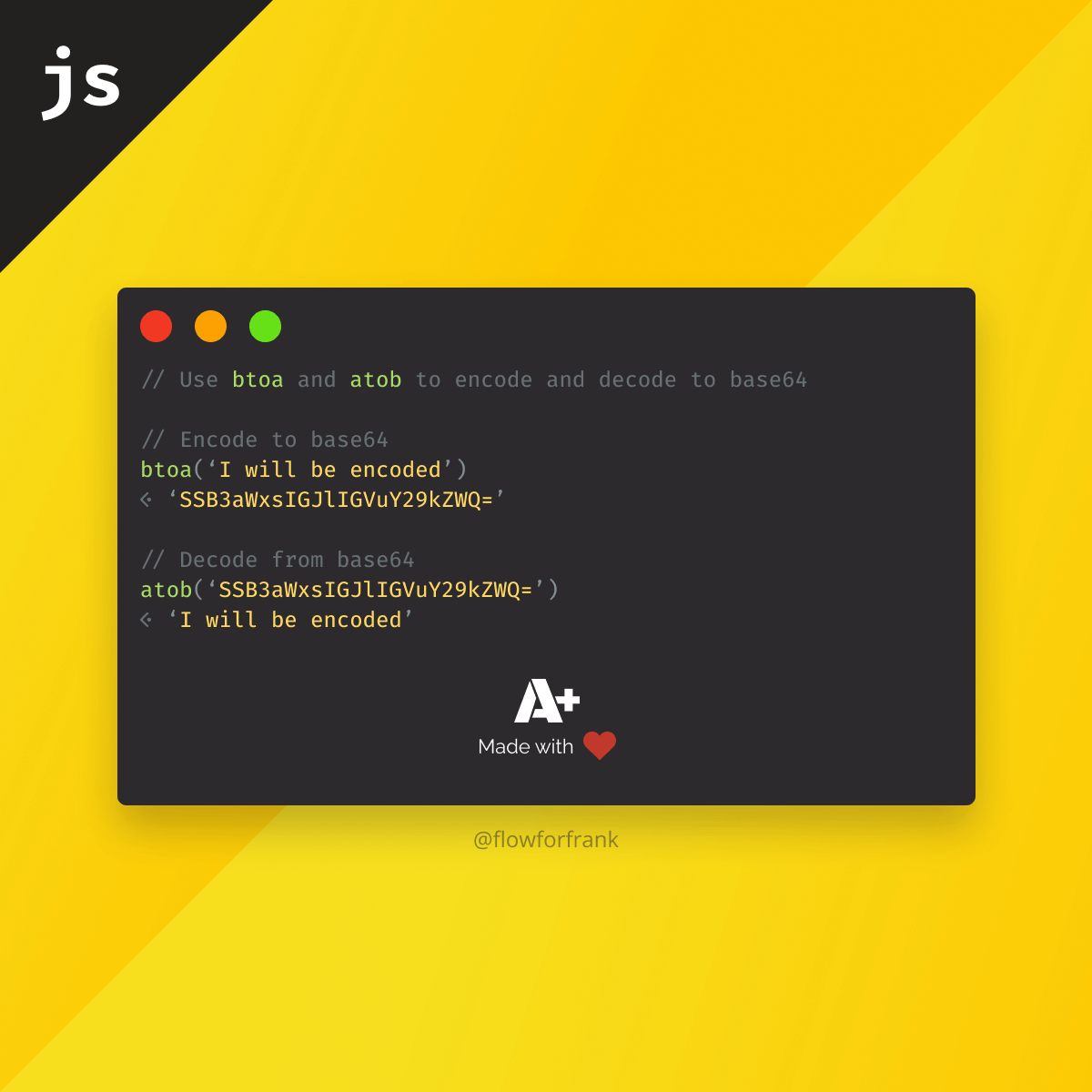
What to Look Out For When Encoding to Base64 in JavaScript
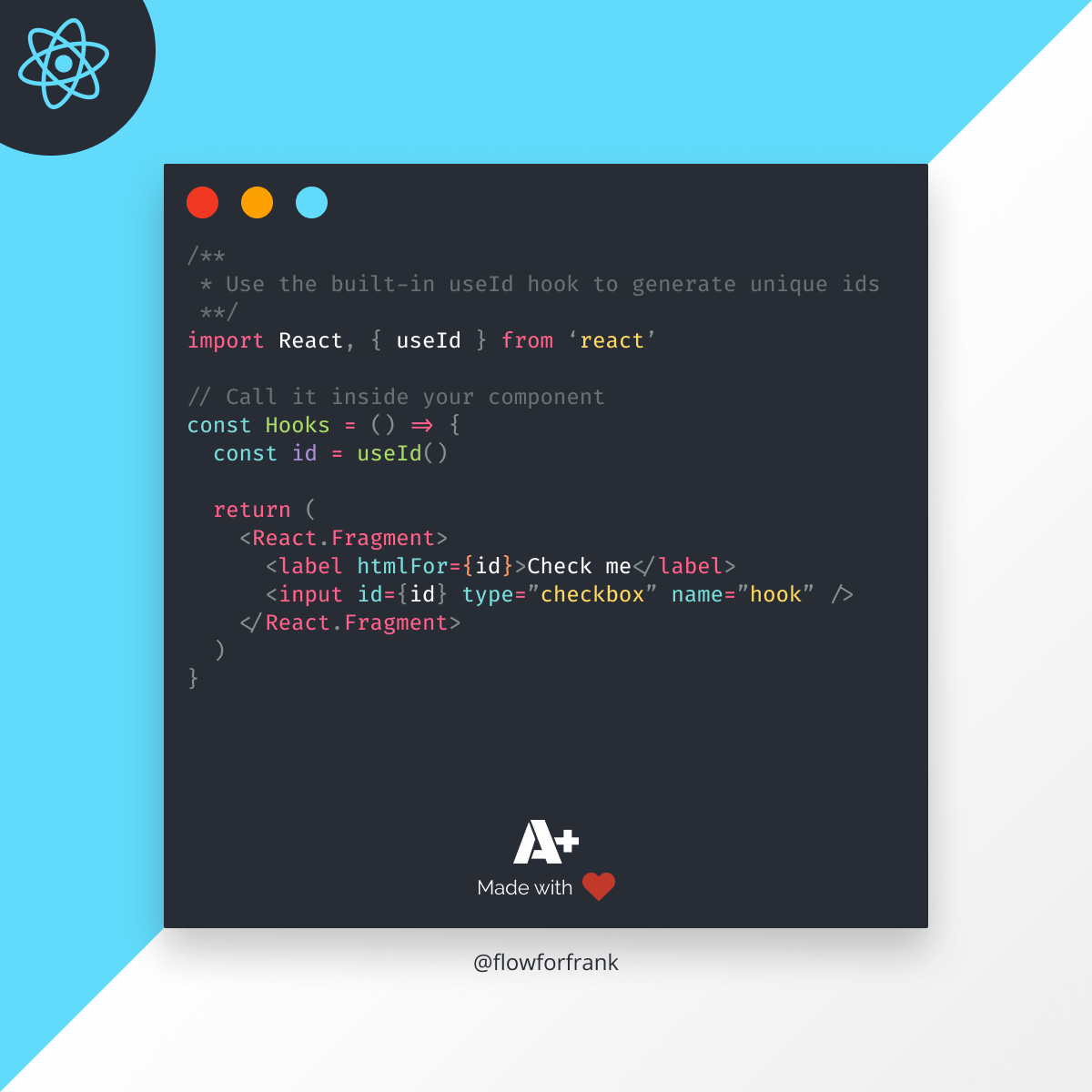
Understanding How to Use the useId Hook in React 18
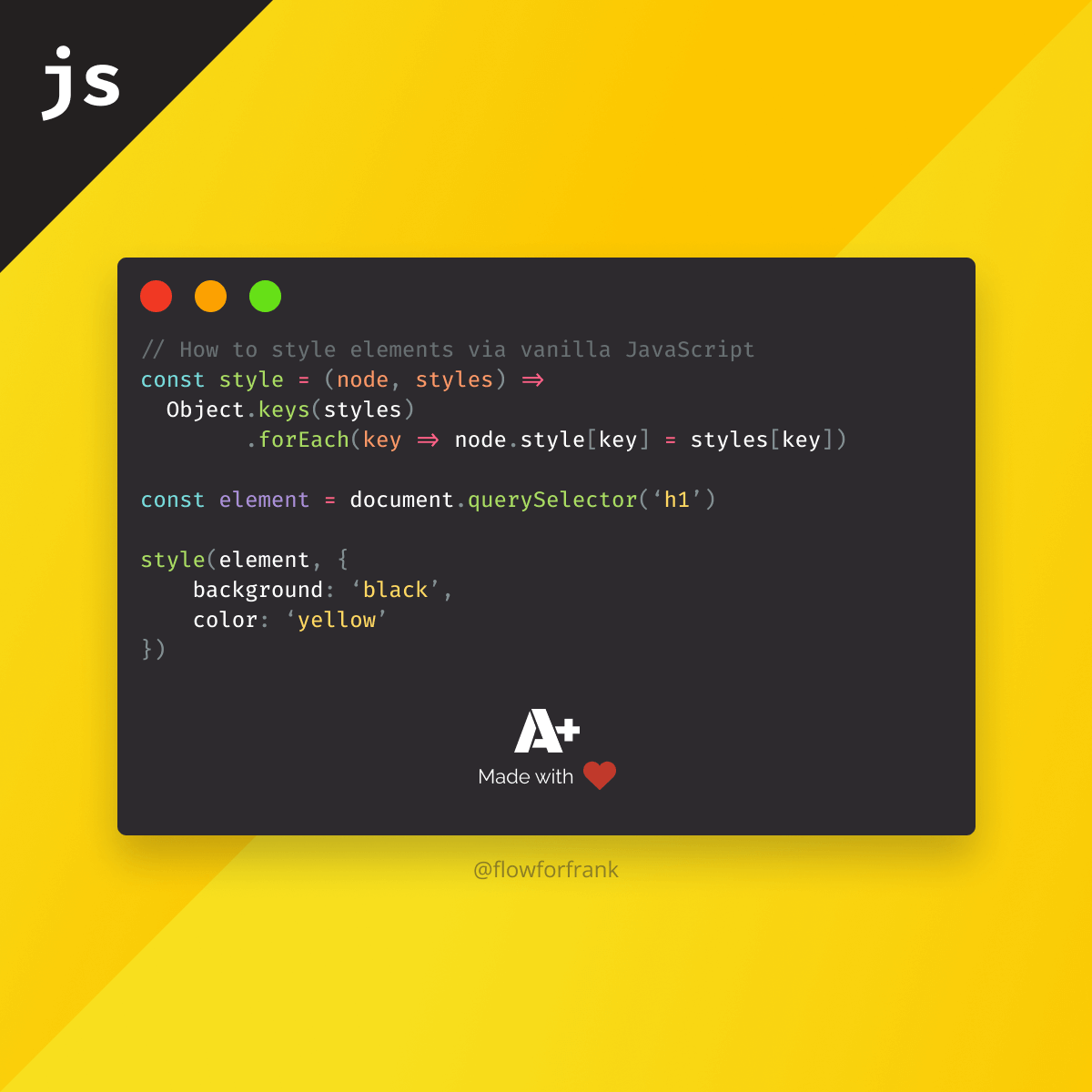