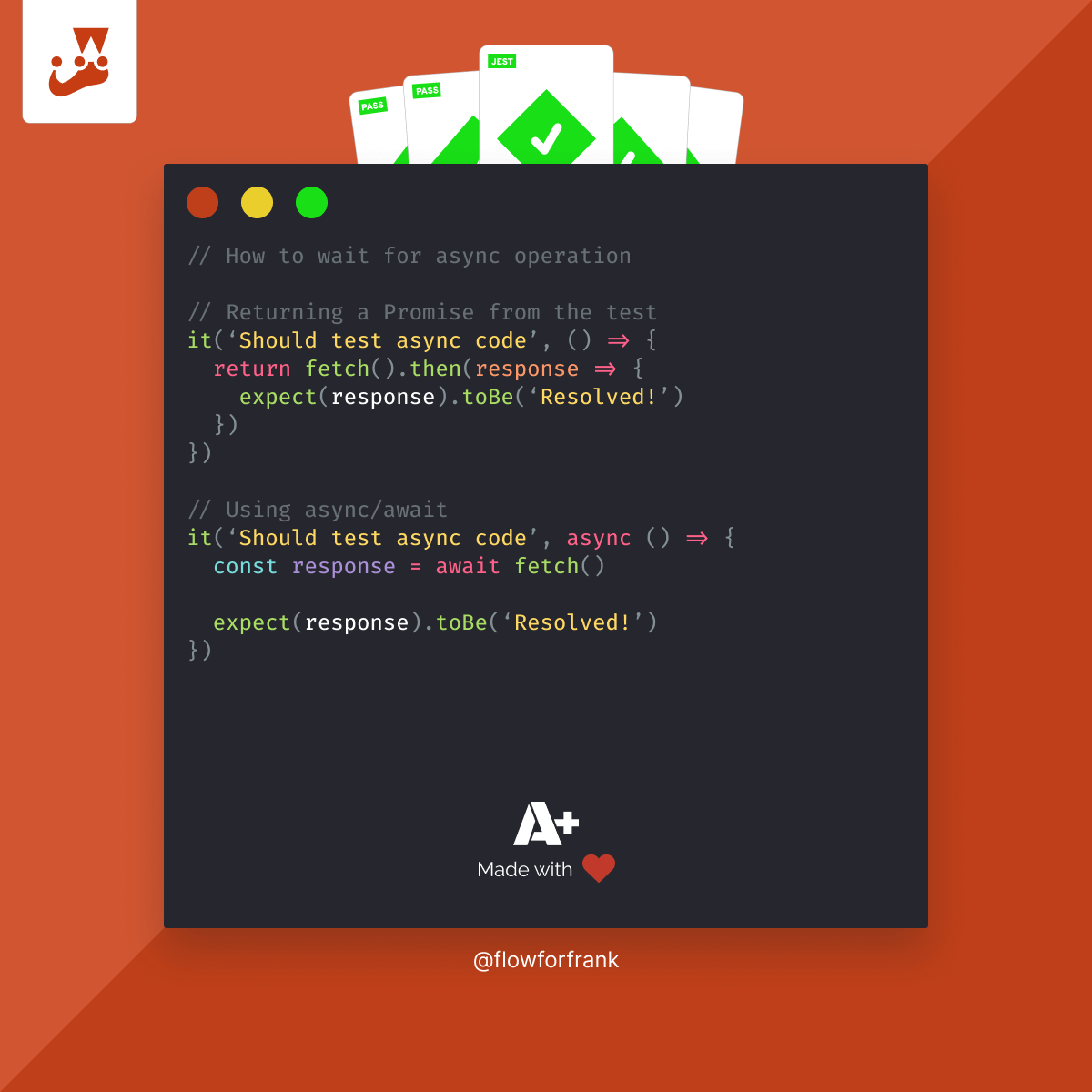
How to Property Wait in Jest for Async Code to Finish
If you need to wait in Jest for x seconds, you likely want to make your test pass that depends on asynchronicity. You should never wait for a fixed time in your tests as they are making your tests non-deterministic, and also increases the time it takes to run your test suite by a fixed amount. Instead, you can do one of the following.
Returning a Promise from the test
Jest is capable of waiting for promises to be resolved or rejected if you return them from your test case. Be warned that if the promise is rejected, the test will fail. You can then write your expect
statements inside the then
callback like so:
it('Should test async code', () => {
return fetch().then(response => {
expect(response).toBe('Resolved!')
})
})
Using async/await
You can also use the async/await
keyword to simplify your code. Here, you don't need a then
callback, but make sure you make your callback function async
in order to use the await
keyword inside it.
it('Should test async code', async () => {
const response = await fetch()
expect(response).toBe('Resolved!')
})
You can also test rejects by introducing a try-catch
block and writing your expect
statements into your catch
block. This way, you can test failures without actually making your tests fail.
it('Should test rejections', async () => {
try {
await fetch()
} catch (error) {
expect(error).toBe('Error!')
}
})

Expecting resolves and rejections
It's also possible to test what is the resolved or rejected values of a promise. For this, you can call either resolves
or rejects
on expect, where a promise is passed.
it('Should test the resolved and rejected value', async () => {
await expect(fetch()).resolves.toBe('Resolved!')
await expect(fetch()).rejects.toMatch('Error!')
})
Mocking Promises
Lastly, you can also mock promises in Jest to ensure they return with a given value. For this, you can use mockResolvedValue
or mockResolvedValueOnce
. Want to know what is the difference between the two? Check out the following webtip:
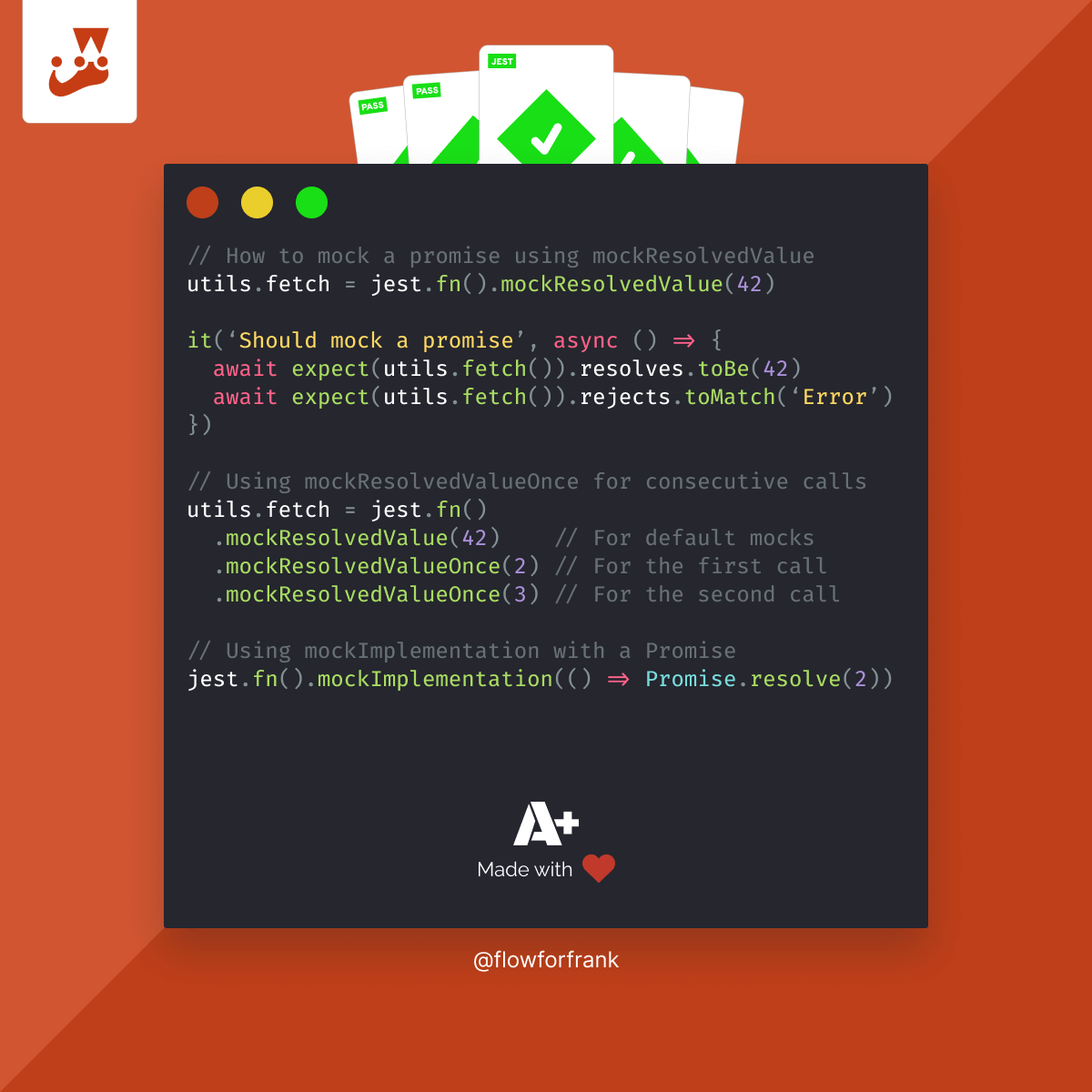
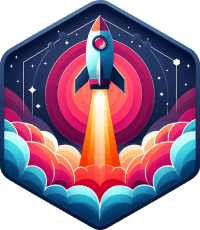
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

JavaScript Unit Testing

Unit Testing for TypeScript and Node.js Developers
