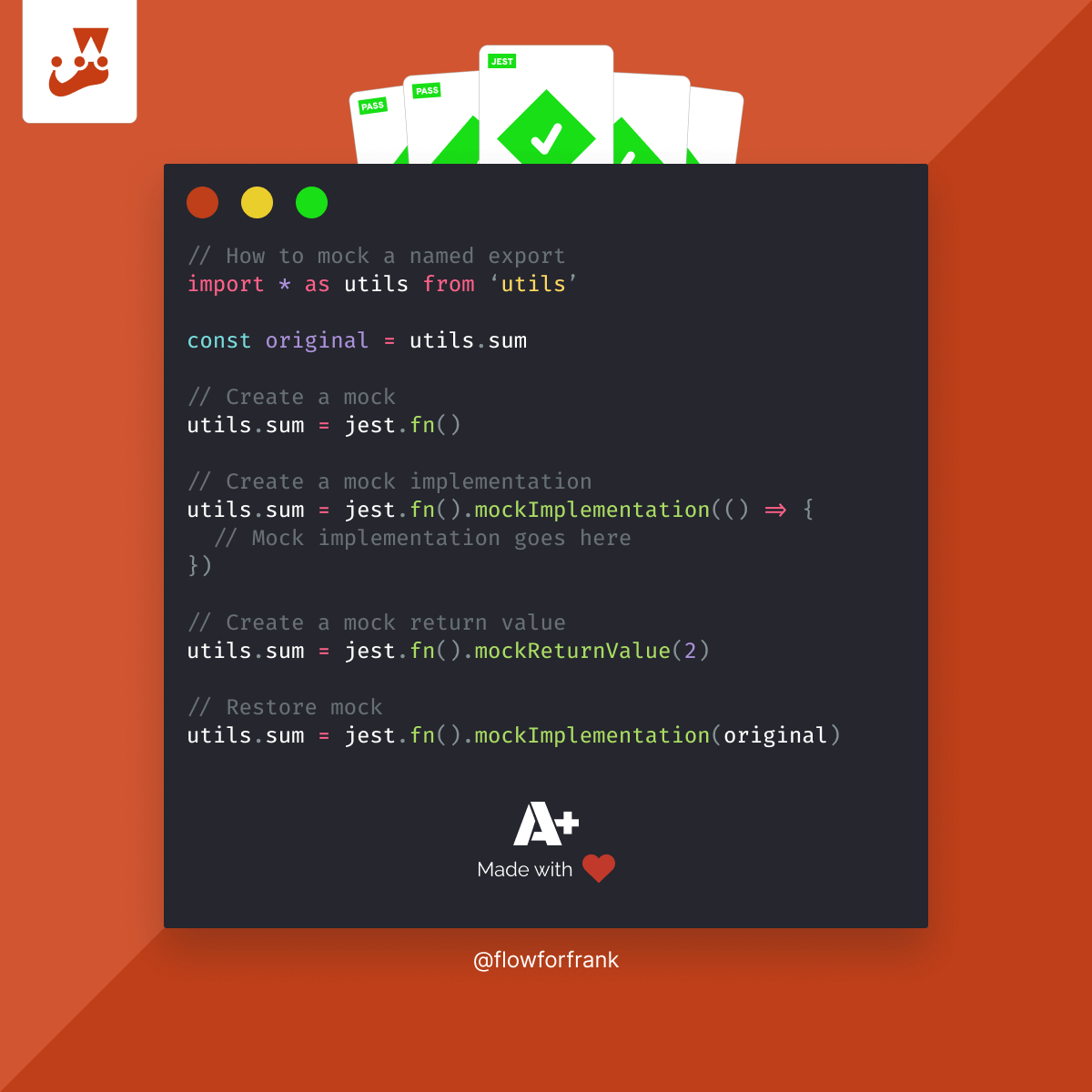
3 Ways to Mock Named Exports in Jest
In order to mock named exports in Jest, you need to import all named exports from a file with an *
and assign jest.fn
to the method that needs to be mocked.
import * as utils from 'utils'
utils.sum = jest.fn()
We can use an alias for the import so that we can access all named exports within an object. Now we can simply reassign one of the methods of utils
to a mock function using jest.fn
. We can also mock the implementation using mockImplementation
:
import * as utils from 'utils'
const original = utils.sum
utils.sum = jest.fn().mockImplementation(() => {
// Mock implementation goes here
})
// Restore mock
utils.sum = jest.fn().mockImplementation(original)
mockImplementation
expects a callback function to be passed that describes the implementation. In case you need to restore the mock later on, we can save the original function into a variable, and call mockImplementation
again with the original function.
You may also simply want to mock only the return value instead of the whole implementation. In order to mock a return value in Jest, we can use mockReturnValue
.
import * as utils from 'utils'
utils.sum = jest.fn().mockReturnValue(2)
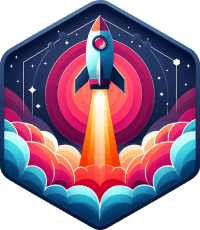
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

JavaScript Unit Testing

Unit Testing for TypeScript and Node.js Developers
