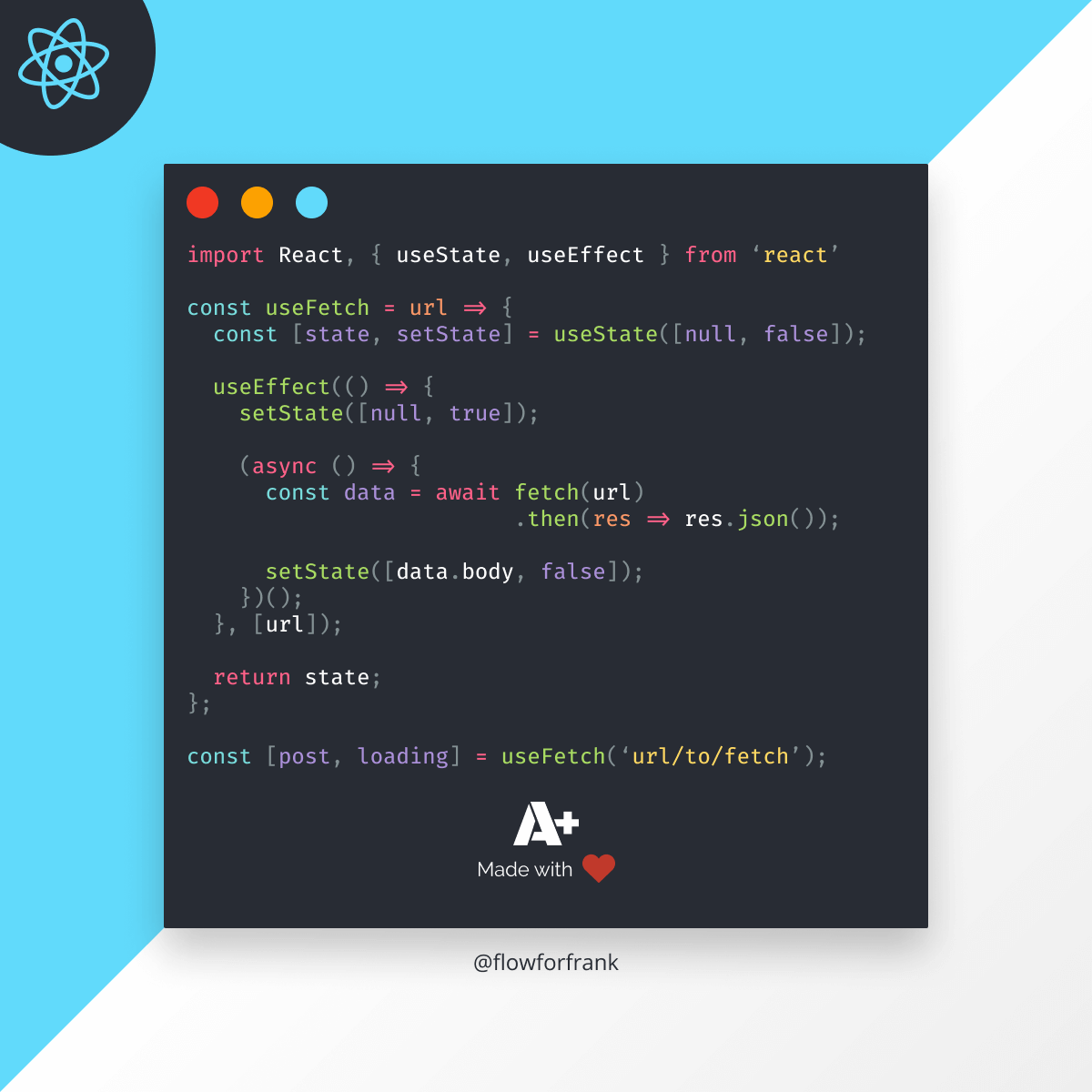
How to Fetch Data With React Hooks
To fetch data from your API using React hooks, you can introduce a custom useFetch
hook that uses useState
and useEffect
internally:
import { useState, useEffect } from 'react'
const useFetch = url => {
const [state, setState] = useState([null, false]);
useEffect(() => {
setState([null, true]);
(async () => {
const data = await fetch(url)
.then(res => res.json());
setState([data.body, false]);
})();
}, [url]);
return state;
};
export default useFetch;
This function returns an array with two variables: A post
object, and a loading
flag. By default, on line:4, you can see that we start out with null
and false
. There's nothing loading. But then we set loading
to true on line:7, and inside an anonymous async
function, we fetch the result. Once the result comes back, we can populate it into the post
variable that is returned.
Note that you can't make the callback of useEffect
async
directly. Therefore, you have to define a sepearate async
function inside it. And of course, once the data is returned, we can set the loading
state back to false. You can pass the url
into the dependency array of the useEffect
. This tells React to only rerun the effect when the URL changes. To use this hook, you can introduce it in your component the following way:
// After importing the useFetch hook
const Post = () => {
const [post, loading] = useFetch('https://api.app.io/post/1');
if (loading) {
return <Loading />
}
return <PostBody content={post} />;
};
If you would like to see it in action, give it a try on Codesandbox

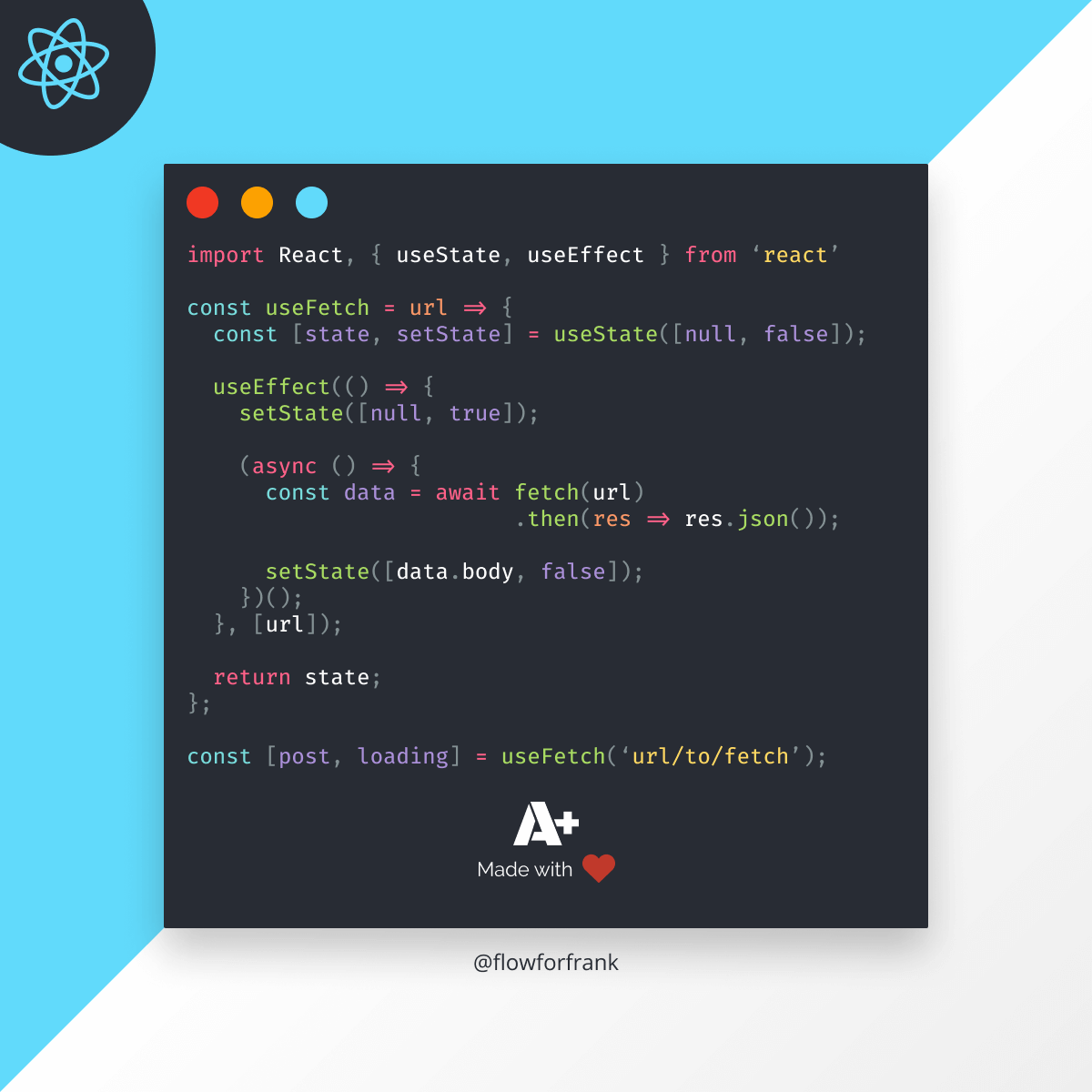
If you are interested in reading more about React hooks, see more examples for custom hooks or just learn about the basics, make sure to check out the article below.
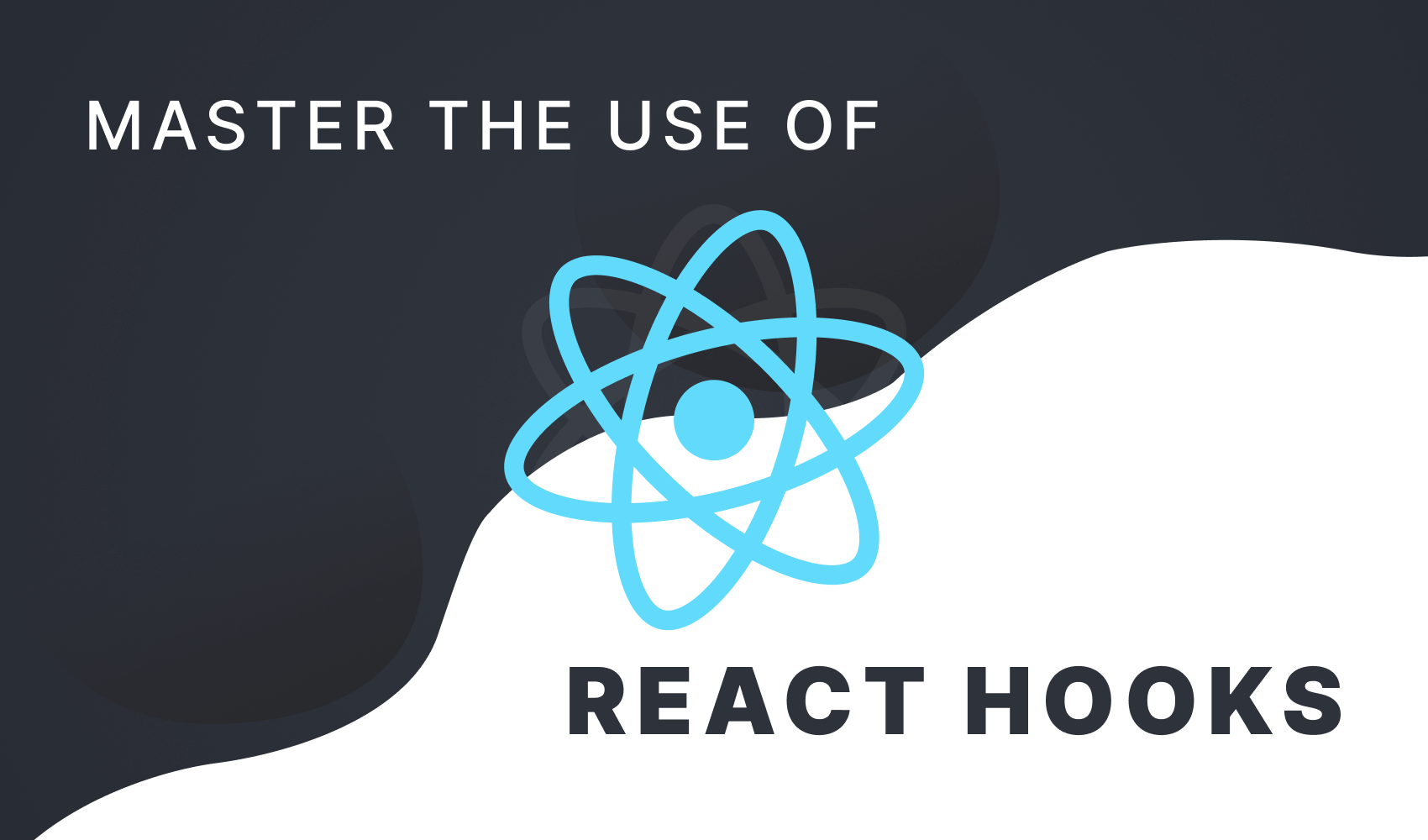
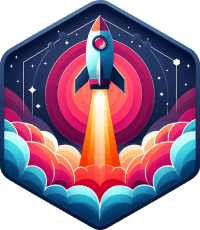
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: